Swift String Exercises: Create a new string without the first and last character of a given string. The string may be any length, including 0
Write a Swift program to create a new string without the first and last character of a given string. The string may be any length, including 0.
Pictorial Presentation:
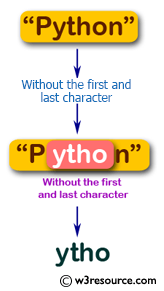
Sample Solution:
Swift Code:
import Foundation
func without_first_last(_ str: String) -> String {
var start_index = str.startIndex
var end_index = str.endIndex
if start_index != end_index
{
start_index = str.index(after: start_index)
end_index = str.index(before: end_index)
}
if start_index < end_index
{
let range = Range(uncheckedBounds: (start_index, end_index))
return str.substring(with: range)
}
else
{
return ""
}
}
print(without_first_last("Python"))
print(without_first_last(" "))
print(without_first_last("Py"))
print(without_first_last("Java"))
Sample Output:
<Swift> ytho av
Go to:
PREV : Write a Swift program to move the last two characters of a given string to the start. The given string length must be at least 2.
NEXT : Write a Swift program to create a new string taking the middle two characters of a given string of even length. The given string length must be at least 2.
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?