Swift String Exercises: Return 'abc' or 'xyz' if a given string begins with 'abc' or 'xyz' otherwise return the empty string
Write a Swift program to return 'abc' or 'xyz' if a given string begins with 'abc' or 'xyz' otherwise return the empty string.
Pictorial Presentation:
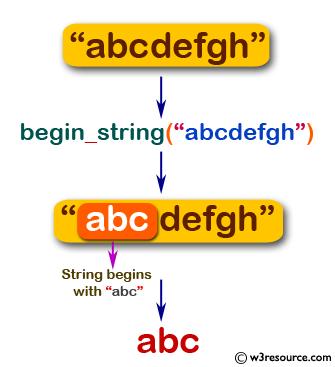
Sample Solution:
Swift Code:
import Foundation
func begin_string(_ str1:String) -> String {
if str1.hasPrefix("abc")
{
return String(str1.characters.prefix(3))
}
else if str1.hasPrefix("xyz")
{
return String(str1.characters.prefix(3))
}
else
{
return ""
}
}
print(begin_string("abcdefgh"))
print(begin_string("wxyz"))
Sample Output:
abc
Go to:
PREV : Write a Swift program to create a new string of any length from a given string where the last two characters are swapped, so 'abcde' will be 'abced'.
NEXT : Write a Swift program to check if the first two characters are same of the last two characters of a given string.
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?