Swift String Exercises: Check if the first or last characters are 'a' of a given string, return the given string without those 'a' characters
Write a Swift program to check if the first or last characters are 'a' of a given string, return the given string without those 'a' characters, and otherwise return the given string.
Pictorial Presentation:
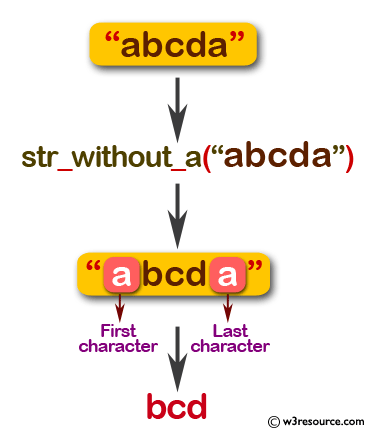
Sample Solution:
Swift Code:
import Foundation
func str_without_a(_ str1:String) -> String {
var new_str = str1
if new_str.hasPrefix("a")
{
new_str.characters.removeFirst()
}
if new_str.hasSuffix("a")
{
new_str.characters.removeLast()
}
return new_str
}
print(str_without_a("abcda"))
print(str_without_a("bcd"))
Sample Output:
bcd bcd
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?