Swift String Exercises: Create a string made of two copies of the last two characters of a given string
Write a Swift program to create a string made of two copies of the last two characters of a given string. The given string length must be at least 2.
Pictorial Presentation:
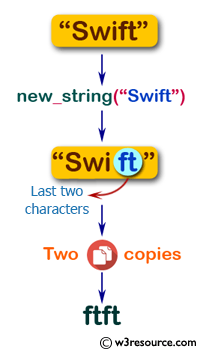
Sample Solution:
Swift Code:
import Foundation
func new_string(_ str: String) -> String {
var result = ""
var last_two_index = str.endIndex
for _ in 0..<2 {
last_two_index = str.index(before: last_two_index)
}
let last_2_chars = str.substring(from: last_two_index)
for _ in 0..<2 {
result.append(last_2_chars)
}
return result
}
print(new_string("Swift"))
print(new_string("Python"))
print(new_string("Java"))
Sample Output:
ftft onon vava
Go to:
PREV : Write a Swift program to insert a given string to another given string where the second string will be in the middle of the first string.
NEXT : Write a Swift program to create a new string made of a copy of the first two characters of a given string. If the given string is shorter than length 2, return whatever there is.
Swift Programming Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?