C Exercises: Insert New value in the array (sorted list )
13. Insert in Sorted Array
Write a program in C to insert the values in the array (sorted list).
The task is to write a C program that inserts a new value into an already sorted array while maintaining the sorted order. The program should prompt the user with the number of elements to input, elements in ascending order, and the value to be inserted. It should then display the array before and after insertion.
Visual Presentation:
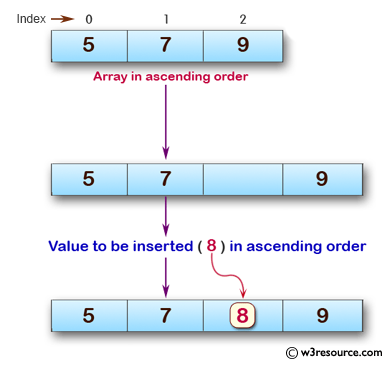
Sample Solution:
C Code:
#include <stdio.h>
#define MAX_SIZE 100
int main() {
int arr1[MAX_SIZE + 1], i, n, p, inval;
// Prompt user for input
printf("\n\nInsert New value in the sorted array:\n");
printf("-----------------------------------------\n");
printf("Input number of elements you want to insert (max %d): ", MAX_SIZE);
scanf("%d", &n);
// Check if the input size exceeds the maximum allowed size
if (n > MAX_SIZE) {
printf("The size of the array cannot exceed %d. Please try again.\n", MAX_SIZE);
return 1; // Exit the program with an error code
}
// Input sorted elements for the array
printf("Input %d elements in the array in ascending order:\n", n);
for (i = 0; i < n; i++) {
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
}
// Input the value to be inserted
printf("Input the value to be inserted : ");
scanf("%d", &inval);
// Display the existing array
printf("The existing array list is :\n");
for (i = 0; i < n; i++) {
printf("% 5d", arr1[i]);
}
// Determine the position where the new value will be inserted
for (i = 0; i < n; i++) {
if (inval < arr1[i]) {
p = i;
break;
} else {
p = i + 1;
}
}
// Move all data at the right side of the array to make space
for (i = n; i >= p; i--) {
arr1[i + 1] = arr1[i];
}
// Insert the new value at the proper position
arr1[p] = inval;
// Display the array after insertion
printf("\n\nAfter Insert the list is :\n");
for (i = 0; i <= n; i++) {
printf("% 5d", arr1[i]);
}
printf("\n");
return 0;
}
Output:
Input number of elements you want to insert (max 100): 5 Input 5 elements in the array in ascending order: element - 0 : 2 element - 1 : 3 element - 2 : 4 element - 3 : 7 element - 4 : 8 Input the value to be inserted : 5 The existing array list is : 2 3 4 7 8 After Insert the list is : 2 3 4 5 7 8
Explanation:
- Include Standard I/O Library: #include <stdio.h>
- Define Maximum Array Size: #define MAX_SIZE 100
- Main Function: int main() { ... }
- Variable Declaration: int arr1[MAX_SIZE + 1], i, n, p, inval;
- Prompt for Input: Display messages prompting the user to insert a new value into the sorted array.
- Input Array Size: Read the size of the array (n) from user input.
- Check Array Size: If n exceeds MAX_SIZE, print an error message and exit.
- Input Array Elements: Read n sorted elements into the array arr1.
- Input Value to Insert: Read the new value to be inserted ('inval').
- Display Existing Array: Prints the existing array elements.
- Determine Insertion Position: Find the position (p) where the 'inval' should be inserted to keep the array sorted.
- Shift Elements Right: Shift elements to the right from the determined position to make space for the new value.
- Insert New Value: Insert 'inval' at the position 'p'.
- Display Updated Array: Prints the array elements after insertion.
- Return Statement: End the program with return 0;.
Flowchart:
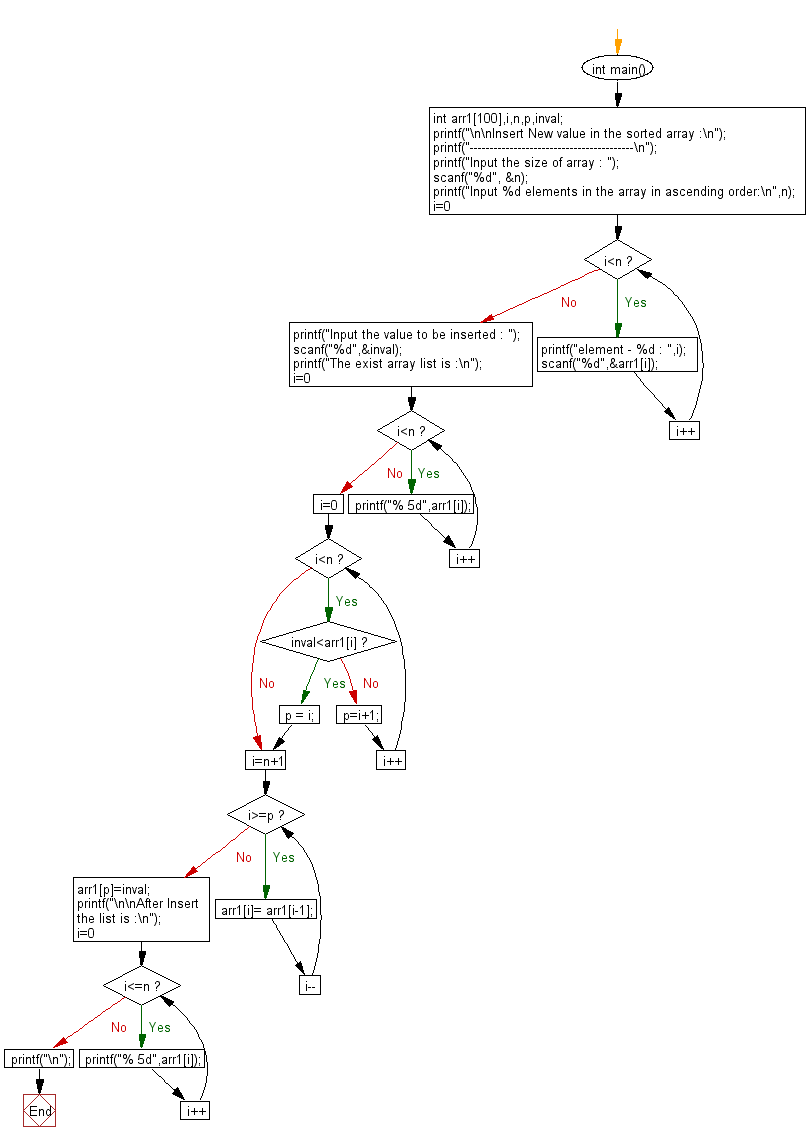
For more Practice: Solve these Related Problems:
- Write a C program to insert a new element into a sorted array using binary search for the correct position.
- Write a C program to insert multiple values into a sorted array and maintain the sorted order.
- Write a C program to insert an element into a sorted array using recursion.
- Write a C program to insert a value into a sorted array and then remove any duplicates.
C Programming Code Editor:
Previous: Write a program in C to sort elements of an array in descending order.
Next: Write a program in C to insert New value in the array (unsorted list ).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.