Divide two numbers or print if division isn't possible
Sum all numbers between two integers, excluding multiples of 17
Write a C program to calculate the sum of all numbers not divisible by 17 between two given integer numbers.
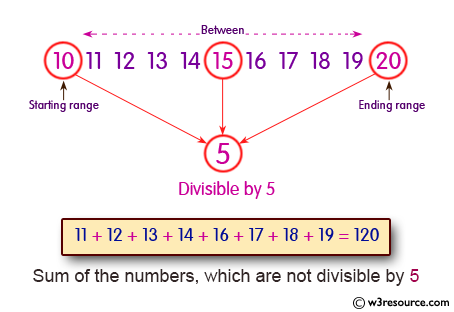
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int x, y, temp, i, sum=0;
// Prompt for user input
printf("\nInput the first integer: ");
scanf("%d", &x);
printf("\nInput the second integer: ");
scanf("%d", &y);
// Swap values if x is greater than y
if(x > y) {
temp = y;
y = x;
x = temp;
}
// Calculate the sum of numbers not divisible by 17
for(i = x; i <= y; i++) {
if((i % 17) != 0) {
sum += i;
}
}
// Display the sum
printf("\nSum: %d\n", sum);
return 0;
}
Sample Output:
Input the first integer: 50 Input the second integer: 99 Sum: 3521
Flowchart:
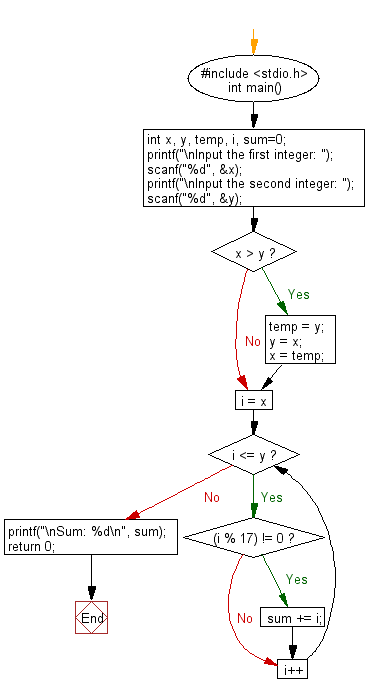
For more Practice: Solve these Related Problems:
- Write a C program to sum all numbers between two given integers, excluding those that are multiples of a user-specified number.
- Write a C program to calculate the sum of all prime numbers between two integers while excluding composite numbers.
- Write a C program to compute the sum of numbers between two integers, excluding even numbers from the total.
- Write a C program to sum numbers in a given range while applying multiple exclusion criteria (e.g., multiples of 17 and multiples of 5).
C Programming Code Editor:
Previous: Write a program that reads two numbers and divide the first number by second number. If the division not possible print "Division not possible".
Next: Write a C program to find all numbers which dividing it by 7 and the remainder is equal to 2 or 3 between two given integer numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.