C Exercises: Swap two numbers without using third variable
Swap two numbers without a temporary variable
Write a C program that swaps two numbers without using a third variable.
This problem requires writing a C program to swap the values of two variables without using a third, temporary variable. The program should demonstrate the swap operation using arithmetic or bitwise XOR operations to achieve the swap directly between the two variables. This approach avoids the need for additional memory allocation, making the solution more efficient in terms of space.
What is swapping in C language?
Swapping in the C language refers to the process of exchanging the values of two variables. This operation involves assigning the value of one variable to another and vice versa, so that each variable holds the initial value of the other. Swapping is a common operation in various algorithms and applications, such as sorting algorithms, to rearrange data.
Applications of Swapping
Swapping is widely used in various programming scenarios, including:
- Sorting Algorithms: Algorithms like bubble sort, quick sort, and others frequently swap elements to arrange data in a particular order.
- Permutations: Generating permutations of a set of values often involves swapping elements.
- Data Manipulation: Various data manipulation tasks require swapping values to achieve desired outcomes.
Visual Presentation:
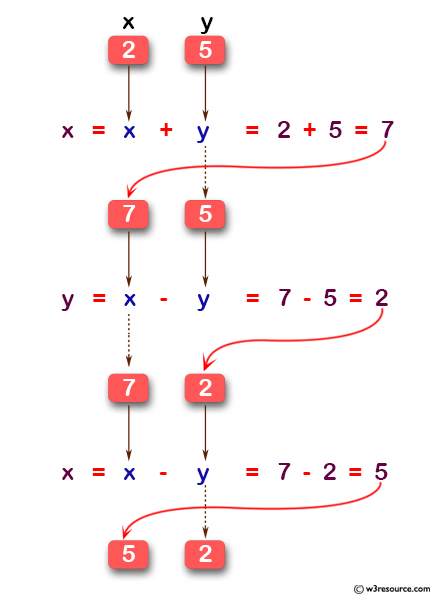
Input value for x & y:
Before swapping the value of x & y: 5 7
After swapping the value of x & y: 7 5
Sample Solution: Using arithmetic operations
C Code:
#include<stdio.h>
int main()
{
int x, y;
printf("Input value for x & y: \n");
scanf("%d%d",&x,&y);
printf("Before swapping the value of x & y: %d %d",x,y);
x=x+y;
y=x-y;
x=x-y;
printf("\nAfter swapping the value of x & y: %d %d",x,y);
return 0;
}
Output:
Input value for x & y: Before swapping the value of x & y: 5 7 After swapping the value of x & y: 7 5
Explanation:
- Include the Standard Input-Output Library: #include<stdio.h> ensures that the functions for input and output operations are available.
- Main Function Definition: int main() begins the execution of the program.
- Variable Declaration: int x, y; declares two integer variables x and y.
- User Input Prompt:
- printf("Input value for x & y: \n"); prompts the user to input values for x and y.
- scanf("%d%d", &x, &y); reads the input values from the user and stores them in x and y.
- Display Initial Values: printf("Before swapping the value of x & y: %d %d", x, y); prints the values of x and y before swapping.
- Swap Values Using Arithmetic Operations:
- x = x + y; adds x and y and stores the result in x.
- y = x - y; subtracts the new value of y (which is the original x + y) by y to get the original value of x and stores it in y.
- x = x - y; subtracts the new value of y (original x) from x to get the original value of y and stores it in x.
- Display Swapped Values: printf("\nAfter swapping the value of x & y: %d %d", x, y); prints the values of x and y after swapping.
- Return Statement: return 0; indicates that the program has executed successfully.
Sample Solution: using bitwise XOR operations
Code:
#include <stdio.h>
int main() {
int x, y;
// Prompt user to input values for x and y
printf("Input value for x & y: \n");
scanf("%d%d", &x, &y);
// Display the values of x and y before swapping
printf("Before swapping the value of x & y: %d %d\n", x, y);
// Swap the values of x and y using bitwise XOR operations
x = x ^ y; // Step 1: x now holds the result of x XOR y
y = x ^ y; // Step 2: y now holds the original value of x
x = x ^ y; // Step 3: x now holds the original value of y
// Display the values of x and y after swapping
printf("After swapping the value of x & y: %d %d\n", x, y);
return 0;
}
Output:
Input value for x & y: 2 3 Before swapping the value of x & y: 2 3 After swapping the value of x & y: 3 2
Explanation:
- Include the Standard Input-Output Library: #include <stdio.h> ensures that the functions for input and output operations are available.
- Main Function Definition: int main() begins the execution of the program.
- Variable Declaration: int x, y; declares two integer variables x and y.
- User Input Prompt:
- printf("Input value for x & y: \n"); prompts the user to input values for x and y.
- scanf("%d%d", &x, &y); reads the input values from the user and stores them in x and y.
- Display Initial Values: printf("Before swapping the value of x & y: %d %d\n", x, y); prints the values of x and y before swapping.
- Swap Values Using Bitwise XOR Operations:
- x = x ^ y; stores the result of x XOR y in x.
- y = x ^ y; stores the original value of x in y by performing x XOR y (since x now holds x XOR y, this operation effectively isolates the original x).
- x = x ^ y; stores the original value of y in x by performing x XOR y (since y now holds the original x).
- Display Swapped Values: printf("After swapping the value of x & y: %d %d\n", x, y); prints the values of x and y after swapping.
- Return Statement: return 0; indicates that the program has executed successfully.
Flowchart:
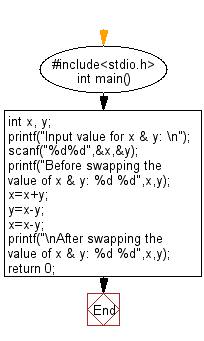
For more Practice: Solve these Related Problems:
- Write a C program to swap two integers using arithmetic operators without a third variable.
- Write a C program to swap two numbers using bitwise XOR operations.
- Write a C program to swap two floating-point numbers without using an extra variable.
- Write a C program to swap two elements in an array without using a temporary variable.
C programming Code Editor:
Previous: Write a C program that accepts a distance in centimeters and prints the corresponding value in inches.
Next: Write a C program to shift given data by two bits to the left.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.