C Exercises: Display firstname, lastname and year of birth sequentially
7. Display Personal Information
Write a program in C that reads a forename, surname and year of birth and displays the names and the year one after another sequentially.
Pictorial Presentation:
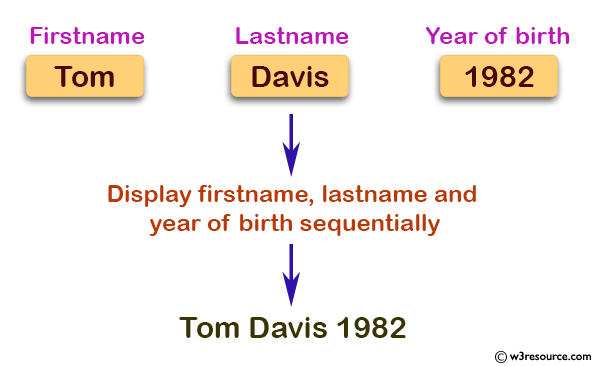
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
int main() {
char firstname[20], lastname[20]; // Declare character arrays for first name and last name with a maximum size of 20 characters each.
int bir_year; // Declare an integer variable 'bir_year' to store the year of birth.
printf("Input your firstname: "); // Prompt the user to input their first name.
scanf("%s", firstname); // Read and store the user's input in 'firstname'.
printf("Input your lastname: "); // Prompt the user to input their last name.
scanf("%s", lastname); // Read and store the user's input in 'lastname'.
printf("Input your year of birth: "); // Prompt the user to input their year of birth.
scanf("%d", &bir_year); // Read and store the user's input in 'bir_year'.
printf("%s %s %d\n", firstname, lastname, bir_year); // Print the first name, last name, and year of birth.
return 0; // Return 0 to indicate successful execution of the program.
}
Sample Output:
Input your firstname: Tom Input your lastname: Davis Input your year of birth: 1982 Tom Davis 1982
Flowchart:
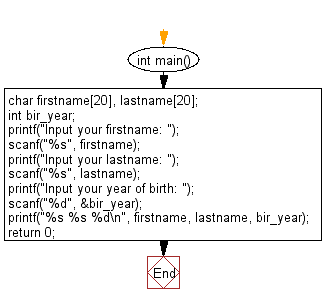
For more Practice: Solve these Related Problems:
- Write a C program to read a first name, last name, and year of birth and then output them in reverse order.
- Write a C program to read a person's forename, surname, and year of birth, calculate the current age, and display a formatted message.
- Write a C program to input a forename, surname, and year of birth, then display only the initials followed by the birth year.
- Write a C program to store personal information in a structure and then print the data in a specified formatted sequence.
C Programming Code Editor:
Previous: Write a program in C that takes minutes as input, and display the total number of hours and minutes.
Next: Write a program in C to calculate the sum of three numbers with getting input in one line separated by a comma.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.