C Exercises: Show a function returning pointer
15. Function Returning a Pointer
Write a C program to demonstrate how a function returns a pointer.
Visual Presentation:
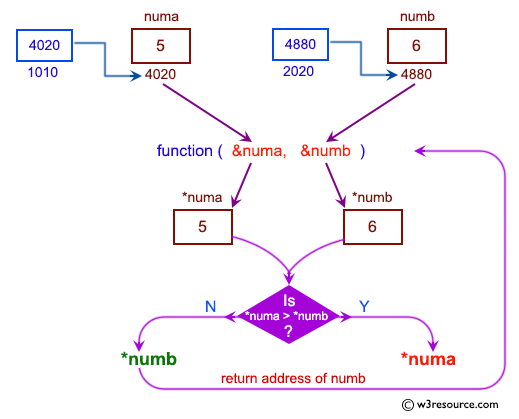
Sample Solution:
C Code:
#include <stdio.h>
// Function prototype declaration
int* findLarger(int*, int*);
int main() {
// Initializing variables
int numa = 0;
int numb = 0;
int *result;
// Displaying the purpose of the program
printf("\n\n Pointer : Show a function returning pointer :\n");
printf("--------------------------------------------------\n");
// Inputting the first number
printf(" Input the first number : ");
scanf("%d", &numa);
// Inputting the second number
printf(" Input the second number : ");
scanf("%d", &numb);
// Calling the function to find the larger number
result = findLarger(&numa, &numb);
// Displaying the larger number found by the function
printf(" The number %d is larger. \n\n", *result);
}
// Function definition to find the larger number
int* findLarger(int *n1, int *n2) {
// Checking which number is larger
if (*n1 > *n2)
return n1; // Return the address of n1 if it's larger
else
return n2; // Return the address of n2 if it's larger
}
Sample Output:
Pointer : Show a function returning pointer : -------------------------------------------------- Input the first number : 5 Input the second number : 6 The number 6 is larger.
Flowchart:
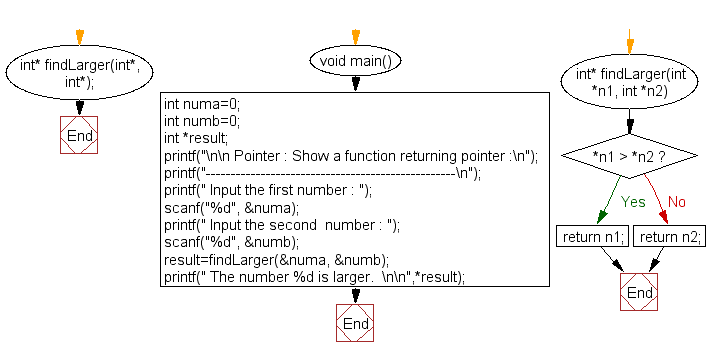
For more Practice: Solve these Related Problems:
- Write a C program where a function returns a pointer to the maximum of two numbers.
- Write a C program in which a function returns a pointer to a dynamically allocated array containing computed results.
- Write a C program to implement a function that returns a pointer to a structure after processing input data.
- Write a C program to write a function that returns a pointer to a string after performing some modifications on it.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to sort an array using Pointer.
Next: Write a program in C to compute the sum of all elements in an array using pointers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.