C Exercises: Sum of all elements in an array
16. Sum of Array Using Pointers
Write a program in C to compute the sum of all elements in an array using pointers.
Visual Presentation:
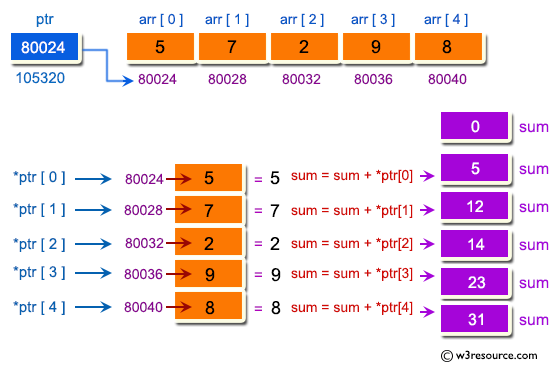
Sample Solution:
C Code:
#include <stdio.h>
int main() {
// Declaring variables
int arr1[10];
int i, n, sum = 0;
int *pt;
// Displaying the purpose of the program
printf("\n\n Pointer : Sum of all elements in an array :\n");
printf("------------------------------------------------\n");
// Inputting the number of elements for the array (maximum 10)
printf(" Input the number of elements to store in the array (max 10) : ");
scanf("%d", &n);
// Inputting elements into the array
printf(" Input %d number of elements in the array : \n", n);
for (i = 0; i < n; i++) {
printf(" element - %d : ", i + 1);
scanf("%d", &arr1[i]);
}
pt = arr1; // pt stores the base address of array arr1
// Calculating the sum of array elements using pointer
for (i = 0; i < n; i++) {
sum = sum + *pt; // Adding the value pointed by pt to the sum
pt++; // Moving the pointer to the next element of the array
}
// Displaying the sum of the array elements
printf(" The sum of array is : %d\n\n", sum);
}
Sample Output:
Pointer : Sum of all elements in an array : ------------------------------------------------ Input the number of elements to store in the array (max 10) : 5 Input 5 number of elements in the array : element - 1 : 2 element - 2 : 3 element - 3 : 4 element - 4 : 5 element - 5 : 6 The sum of array is : 20
Flowchart:
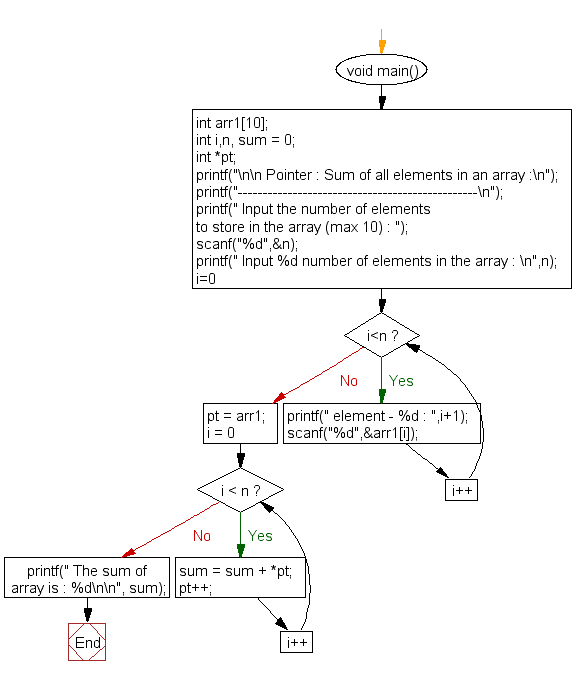
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of an array’s elements using pointer arithmetic instead of indexing.
- Write a C program to calculate the sum of an array using recursion with pointers.
- Write a C program to sum an array of integers dynamically allocated and accessed via pointers.
- Write a C program to find the sum of even-indexed and odd-indexed elements separately using pointers.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to show how a function returning pointer.
Next: Write a program in C to print the elements of an array in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.