C Exercises: Print the elements of an array in reverse order
17. Print Array in Reverse Using Pointer
Write a program in C to print the elements of an array in reverse order.
Visual Presentation:
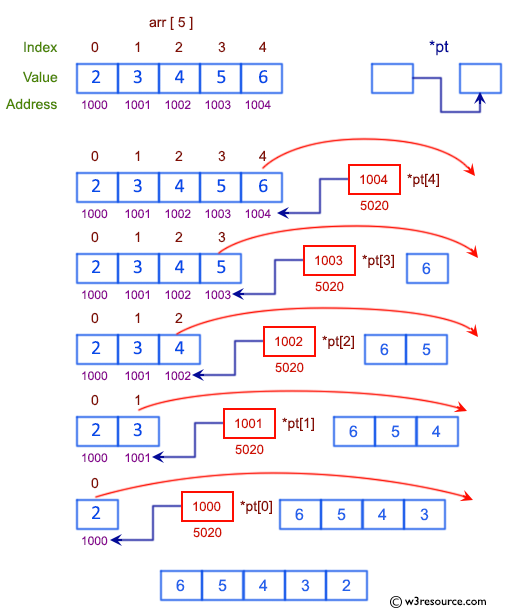
Sample Solution:
C Code:
#include <stdio.h>
int main() {
// Declaring variables
int n, i, arr1[15];
int *pt;
// Displaying the purpose of the program
printf("\n\n Pointer : Print the elements of an array in reverse order :\n");
printf("----------------------------------------------------------------\n");
// Inputting the number of elements for the array (maximum 15)
printf(" Input the number of elements to store in the array (max 15) : ");
scanf("%d", &n);
pt = &arr1[0]; // pt stores the address of base array arr1
// Inputting elements into the array using pointers
printf(" Input %d number of elements in the array : \n", n);
for (i = 0; i < n; i++) {
printf(" element - %d : ", i + 1);
scanf("%d", pt); // Accepting the address of the value
pt++;
}
pt = &arr1[n - 1]; // Setting pt to point at the last element of the array
// Displaying the elements of the array in reverse order using pointers
printf("\n The elements of array in reverse order are :");
for (i = n; i > 0; i--) {
printf("\n element - %d : %d ", i, *pt); // Printing the element and its value
pt--; // Moving the pointer to the previous element
}
printf("\n\n");
}
Sample Output:
Pointer : Print the elements of an array in reverse order : ---------------------------------------------------------------- Input the number of elements to store in the array (max 15) : 5 Input 5 number of elements in the array : element - 1 : 2 element - 2 : 3 element - 3 : 4 element - 4 : 5 element - 5 : 6 The elements of array in reverse order are : element - 5 : 6 element - 4 : 5 element - 3 : 4 element - 2 : 3 element - 1 : 2
Flowchart:
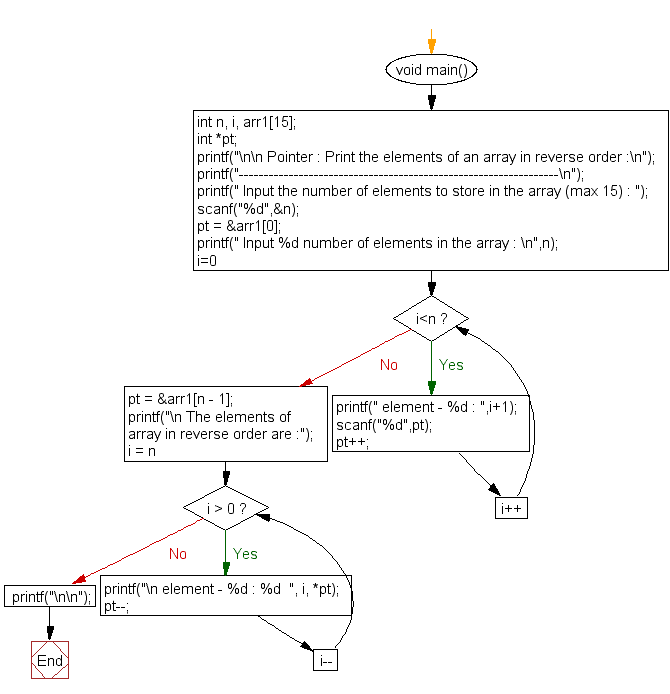
For more Practice: Solve these Related Problems:
- Write a C program to print an array in reverse order using pointer decrement without using a loop index.
- Write a C program to display an array in reverse order recursively using pointer arithmetic.
- Write a C program to input an array and then print its elements in reverse order using a for loop with pointers.
- Write a C program to reverse the array in place using pointers and then print the modified array.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to compute the sum of all elements in an array using pointers.
Next: Write a program in C to show the usage of pointer to structure.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.