C Exercises: Show the usage of pointer to structure
C Pointer : Exercise-18 with Solution
Write a program in C to demonstrate the use of pointers to structures.
Visual Presentation:
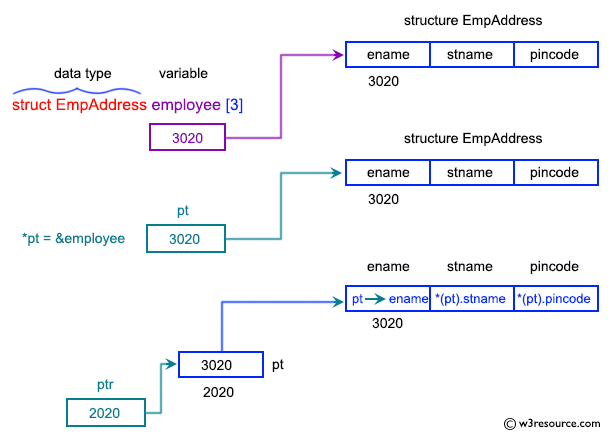
Sample Solution:
C Code:
#include <stdio.h>
// Defining a structure to hold employee address details
struct EmpAddress {
char *ename; // Employee name (string pointer)
char stname[20]; // Street name (array of characters)
int pincode; // Pincode (integer)
}
// Initializing an instance of the structure with data
employee = {"John Alter", "Court Street \n", 654134}, *pt = &employee;
int main() {
// Displaying the purpose of the program
printf("\n\n Pointer : Show the usage of pointer to structure :\n");
printf("--------------------------------------------------------\n");
// Printing employee details using pointer to structure
printf(" %s from %s \n\n", pt->ename, (*pt).stname);
return 0;
}
Sample Output:
Pointer : Show the usage of pointer to structure : -------------------------------------------------------- John Alter from Court Street
Flowchart:
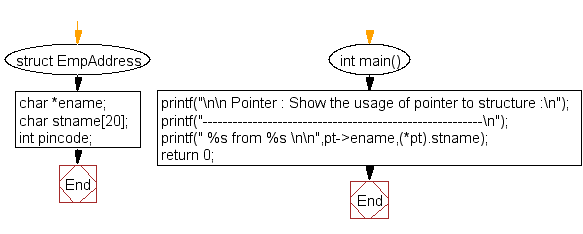
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to print the elements of an array in reverse order.
Next: Write a program in C to show a pointer to union.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/pointer/c-pointer-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics