C Exercises: Print all the alphabets
21. Print Alphabets Using Pointer
Write a program in C to print all the alphabets using pointer.
Visual Presentation:
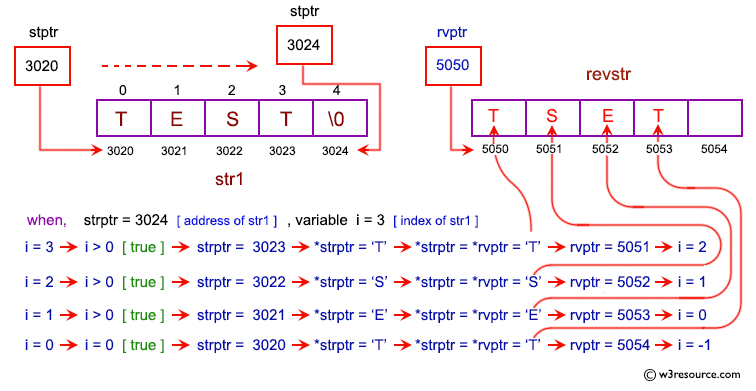
Sample Solution:
C Code:
#include <stdio.h>
int main() {
// Declaration of variables
char alph[27]; // Array to store alphabets
int x;
char *ptr; // Pointer to char
// Displaying the purpose of the program
printf("\n\n Pointer : Print all the alphabets:\n");
printf("----------------------------------------\n");
ptr = alph; // Assigning the base address of array 'alph' to pointer 'ptr'
// Storing alphabets in the array using pointer arithmetic
for (x = 0; x < 26; x++) {
*ptr = x + 'A'; // Assigning ASCII values of alphabets to the array through the pointer
ptr++; // Moving the pointer to the next memory location
}
ptr = alph; // Resetting the pointer to the base address of array 'alph'
// Printing the stored alphabets using the pointer
printf(" The Alphabets are : \n");
for (x = 0; x < 26; x++) {
printf(" %c ", *ptr); // Printing each alphabet
ptr++; // Moving the pointer to the next memory location
}
printf("\n\n");
return 0;
}
Sample Output:
Pointer : Print all the alphabates: ---------------------------------------- The Alphabates are : A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Flowchart:
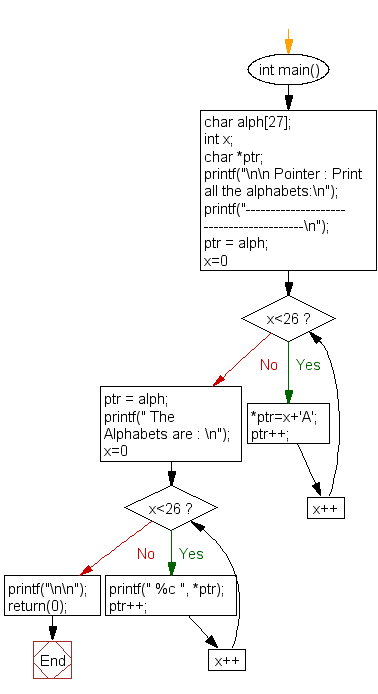
For more Practice: Solve these Related Problems:
- Write a C program to print all uppercase alphabets using pointer arithmetic and a loop.
- Write a C program to store the alphabets in an array and print them in reverse order using a pointer.
- Write a C program to print the alphabets by incrementing a pointer through a string literal.
- Write a C program to print both uppercase and lowercase alphabets using pointers and conditional statements.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to show a pointer to an array which contents are pointer to structure.
Next: Write a program in C to print a string in reverse using a pointer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.