C Exercises: Convert a string to double
4. String to Double Conversion
Write a C program to convert a string to a double.
Sample Solution:
C Code:
#include<stdio.h> // Include the standard input/output header file.
#include<stdlib.h> // Include the standard library header file.
int main () // Start of the main function.
{
char input[] = "8.0 2.0"; // Declare a character array 'input' and initialize it with "8.0 2.0".
char * ptr_end; // Declare a pointer to char 'ptr_end'.
double x, y; // Declare two double variables 'x' and 'y'.
x = strtod (input,&ptr_end); // Convert the first part of 'input' to a double, store in 'x', and update 'ptr_end'.
y = strtod (ptr_end,NULL); // Convert the remaining part of 'input' to a double, store in 'y'.
printf ("\nOutput= %.2lf\n\n", x/y); // Print the result of x/y with two decimal places.
return 0; // Return 0 to indicate successful execution of the program.
} // End of the main function.
Sample Output:
Output= 4.00
Flowchart:
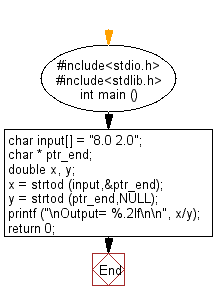
For more Practice: Solve these Related Problems:
- Write a C program to convert a floating-point string in scientific notation to a double using strtod().
- Write a C program to convert a string with leading and trailing whitespace to a double and handle conversion errors.
- Write a C program to convert a string to a double and verify that the conversion consumed the entire string.
- Write a C program to convert a string to a double while handling locale-specific decimal separators.
C Programming Code Editor:
Previous: Write a C program to convert a string to a long integer.
Next: Write a C program to generate a random number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.