C#: Get the absolute value of the difference between two given numbers
Absolute Difference or Double It
Write a C# program to get the absolute value of the difference between two given numbers. Return double the absolute value of the difference if the first number is greater than the second number.
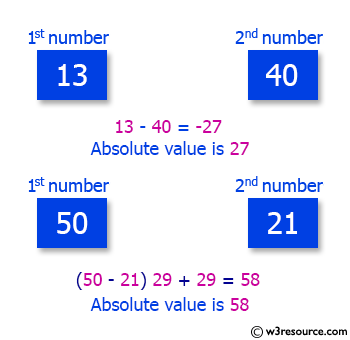
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
// This is the beginning of the Exercise20 class
public class Exercise20 {
// This is the main method where the program execution starts
static void Main(string[] args)
{
// Displaying the result of the 'result' method with different integer arguments
Console.WriteLine(result(13, 40)); // Test case 1: 'a' < 'b', returns 'b - a'
Console.WriteLine(result(50, 21)); // Test case 2: 'a' > 'b', returns '(a - b) * 2'
Console.WriteLine(result(0, 23)); // Test case 3: 'a' < 'b', returns 'b - a'
}
// Method to calculate and return a result based on two integer inputs
public static int result(int a, int b)
{
if (a > b)
{
// If 'a' is greater than 'b', return the difference of 'a' and 'b' multiplied by 2
return (a - b) * 2;
}
// If 'a' is not greater than 'b', return the difference of 'b' and 'a'
return b - a;
}
}
Sample Output:
27 58 23
Flowchart:
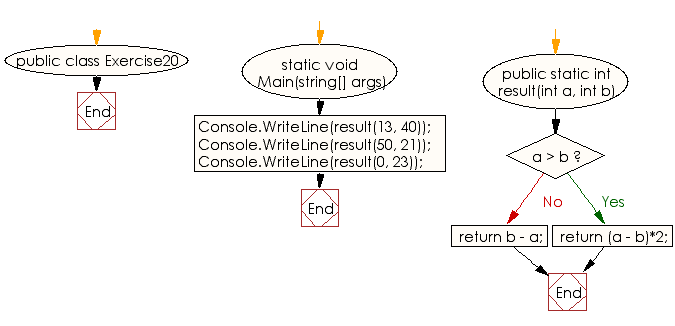
For more Practice: Solve these Related Problems:
- Write a C# program that returns the square of the absolute difference between two numbers.
- Write a C# program to return double the absolute difference only if both numbers are prime.
- Write a C# program that returns the cube of the difference if the result is even.
- Write a C# program that returns the absolute difference unless one of the numbers is zero, in which case return -1.
Go to:
PREV : Sum or Triple Sum of Integers.
NEXT : Check for 20 or Sum Equals 20.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.