C#: Check the sum of the two given integers and return true if one of the integer is 20 or if their sum is 20
Check for 20 or Sum Equals 20
Write a C# program to check the sum of the two given integers. Return true if one of the integers is 20 or if their sum is 20.
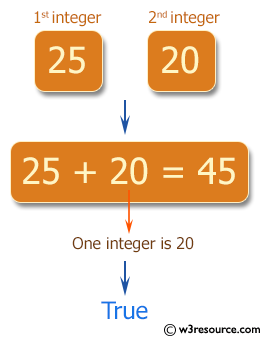
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
// This is the beginning of the Exercise21 class
public class Exercise21 {
// This is the main method where the program execution starts
static void Main(string[] args)
{
int x, y; // Declaring variables x and y to store integers
int result; // Declaring a variable result (not used in the provided code snippet)
Console.WriteLine("\nInput an integer:"); // Prompting the user to input an integer
x = Convert.ToInt32(Console.ReadLine()); // Reading the first integer input provided by the user
Console.WriteLine("Input another integer:"); // Prompting the user to input another integer
y = Convert.ToInt32(Console.ReadLine()); // Reading the second integer input provided by the user
// Checking if x equals 20 OR y equals 20 OR the sum of x and y equals 20
Console.WriteLine(x == 20 || y == 20 || (x + y == 20)); // Outputting the result of the condition evaluation
}
}
Sample Output:
Input an integer: 25 Input another integer: 20 True
Flowchart:
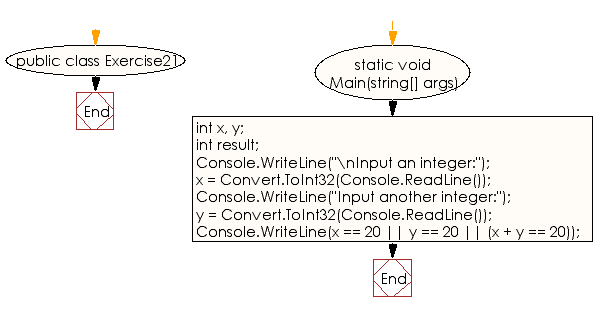
C# Sharp Code Editor:
Previous: Write a C# program to get the absolute value of the difference between two given numbers. Return double the absolute value of the difference if the first number is greater than second number.
Next: Write a C# program to check if an given integer is within 20 of 100 or 200.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.