C#: Check if an given integer is within 20 of 100 or 200
C# Sharp Basic: Exercise-22 with Solution
Check Within 20 of 100 or 200
Write a C# program to check if the given integer is within 20 of 100 or 200.
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
// This is the beginning of the Exercise22 class
public class Exercise22 {
// This is the main method where the program execution starts
static void Main(string[] args) {
Console.WriteLine("\nInput an integer:"); // Prompting the user to input an integer
int x = Convert.ToInt32(Console.ReadLine()); // Reading the integer input provided by the user
Console.WriteLine(result(x)); // Calling the 'result' method and printing the result
}
// Method to check if the given integer is within 20 units of 100 or 200
public static bool result(int n) {
// Checking if the absolute difference between 'n' and 100 is less than or equal to 20
// OR the absolute difference between 'n' and 200 is less than or equal to 20
if (Math.Abs(n - 100) <= 20 || Math.Abs(n - 200) <= 20)
return true; // Return true if the condition is satisfied
return false; // Return false if the condition is not satisfied
}
}
Sample Output:
Input an integer: 25 False
Sample Output:
Input an integer: 50 False
Sample Output:
Input an integer: 70 False
Sample Output:
Input an integer: 80 True
Sample Output:
Input an integer: 90 True
Sample Output:
Input an integer: 110 True
Sample Output:
Input an integer: 120 True
Sample Output:
Input an integer: 125 False
Sample Output:
Input an integer: 130 False
Flowchart:
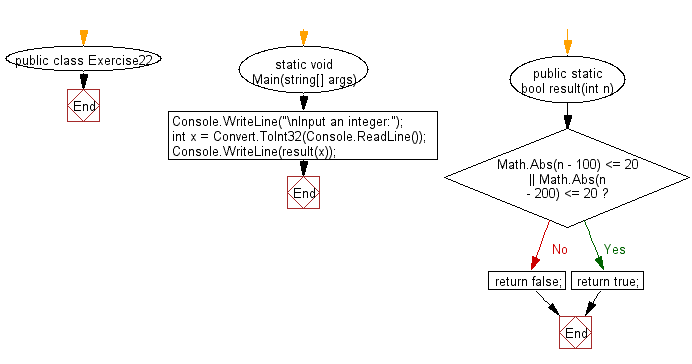
C# Sharp Code Editor:
Previous: Write a C# program to check the sum of the two given integers and return true if one of the integer is 20 or if their sum is 20.
Next: Write a C# program to convert a given string into lowercase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic/csharp-basic-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics