C#: String representation of a date and time to its DateTime equivalent using the specified array
Write a C# Sharp program to convert the specified string representation of a date and time to its DateTime equivalent. This is done using the specified array of formats, culture-specific format information, and style.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
public class Example21
{
public static void Main()
{
// Array of different date formats
string[] formats= {"M/d/yyyy h:mm:ss tt", "M/d/yyyy h:mm tt",
"MM/dd/yyyy hh:mm:ss", "M/d/yyyy h:mm:ss",
"M/d/yyyy hh:mm tt", "M/d/yyyy hh tt",
"M/d/yyyy h:mm", "M/d/yyyy h:mm",
"MM/dd/yyyy hh:mm", "M/dd/yyyy hh:mm"};
// Array of date strings in different formats
string[] dateStrings = {"8/1/2016 6:32 PM", "08/01/2016 6:32:05 PM",
"8/1/2016 6:32:00", "08/01/2016 06:32",
"08/01/2016 06:32:00 PM", "08/01/2016 06:32:00"};
DateTime dateValue; // Variable to store parsed DateTime values
// Iterate through each date string in the array
foreach (string dateString in dateStrings)
{
// Try parsing each date string using various formats and Chinese culture
if (DateTime.TryParseExact(dateString, formats,
new CultureInfo("zh-CN"),
DateTimeStyles.None,
out dateValue))
// Display converted date if successful
Console.WriteLine("Converted '{0}' to {1}.", dateString, dateValue);
else
// Display error message if unable to convert to a date
Console.WriteLine("Unable to convert '{0}' to a date.", dateString);
}
}
}
Sample Output:
Unable to convert '8/1/2016 6:32 PM' to a date. Unable to convert '08/01/2016 6:32:05 PM' to a date. Converted '8/1/2016 6:32:00' to 8/1/2016 6:32:00 AM. Converted '08/01/2016 06:32' to 8/1/2016 6:32:00 AM. Unable to convert '08/01/2016 06:32:00 PM' to a date. Converted '08/01/2016 06:32:00' to 8/1/2016 6:32:00 AM.
Flowchart:
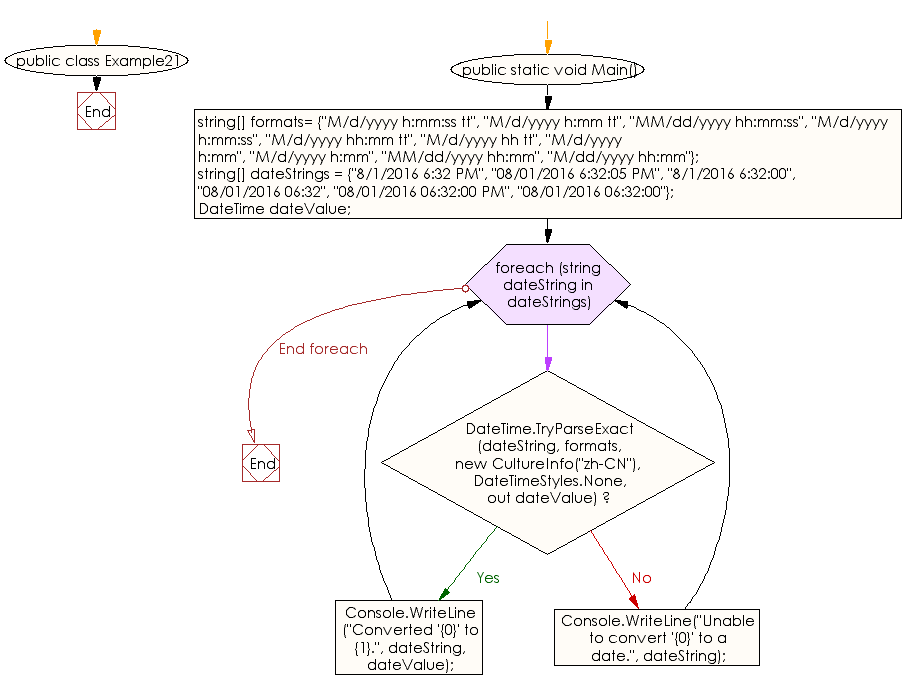
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare DateTime objects.
Next: Write a C# Sharp program which shows that when a time that falls within this range is converted to a long integer value and is then restored and the original value is adjusted to become a valid time.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.