C#: Create a file and write an array of strings
Write a program in C# Sharp to create a file and write an array of strings to the file.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace for basic functionalities
using System.IO; // Importing the System.IO namespace for file operations
class WriteTextFile // Declaring a class named WriteTextFile
{
static void Main() // Declaring the Main method
{
string fileName = @"mytest.txt"; // Initializing a string variable with the file name
string[] ArrLines; // Declaring a string array variable to store lines of text
int n, i; // Declaring variables for counting lines and iterating
Console.Write("\n\n Create a file and write an array of strings :\n"); // Displaying a message in the console
Console.Write("---------------------------------------------------\n");
if (File.Exists(fileName)) // Checking if the file already exists and deleting it if true
{
File.Delete(fileName); // Deleting the file if it exists
}
Console.Write(" Input number of lines to write in the file :"); // Prompting the user to input the number of lines to write
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of lines provided by the user
ArrLines = new string[n]; // Initializing the string array with the provided number of lines
Console.Write(" Input {0} strings below :\n", n); // Prompting the user to input the lines of text
// Loop to input lines of text based on the user-defined count
for (i = 0; i < n; i++)
{
Console.Write(" Input line {0} : ", i + 1); // Prompting for each line
ArrLines[i] = Console.ReadLine(); // Reading and storing each line of text in the array
}
// Writing all lines from the array to the file
System.IO.File.WriteAllLines(fileName, ArrLines);
// Reading and displaying the content of the file
using (StreamReader sr = File.OpenText(fileName)) // Opening a StreamReader to read the content of the file
{
string s = "";
Console.Write("\n The content of the file is :\n"); // Displaying a message
Console.Write("----------------------------------\n");
// Reading and displaying each line of the file
while ((s = sr.ReadLine()) != null) // Looping through each line until the end of the file
{
Console.WriteLine(" {0} ", s); // Displaying each line in the console
}
Console.WriteLine(); // Adding a newline for formatting
}
}
}
Sample Output:
Create a file and write an array of strings : --------------------------------------------------- Input number of lines to write in the file :2 Input 2 strings below : Input line 1 : This is a 1st line Input line 2 : This is a 2nd line The content of the file is : ---------------------------------- This is a 1st line This is a 2nd line
Flowchart :
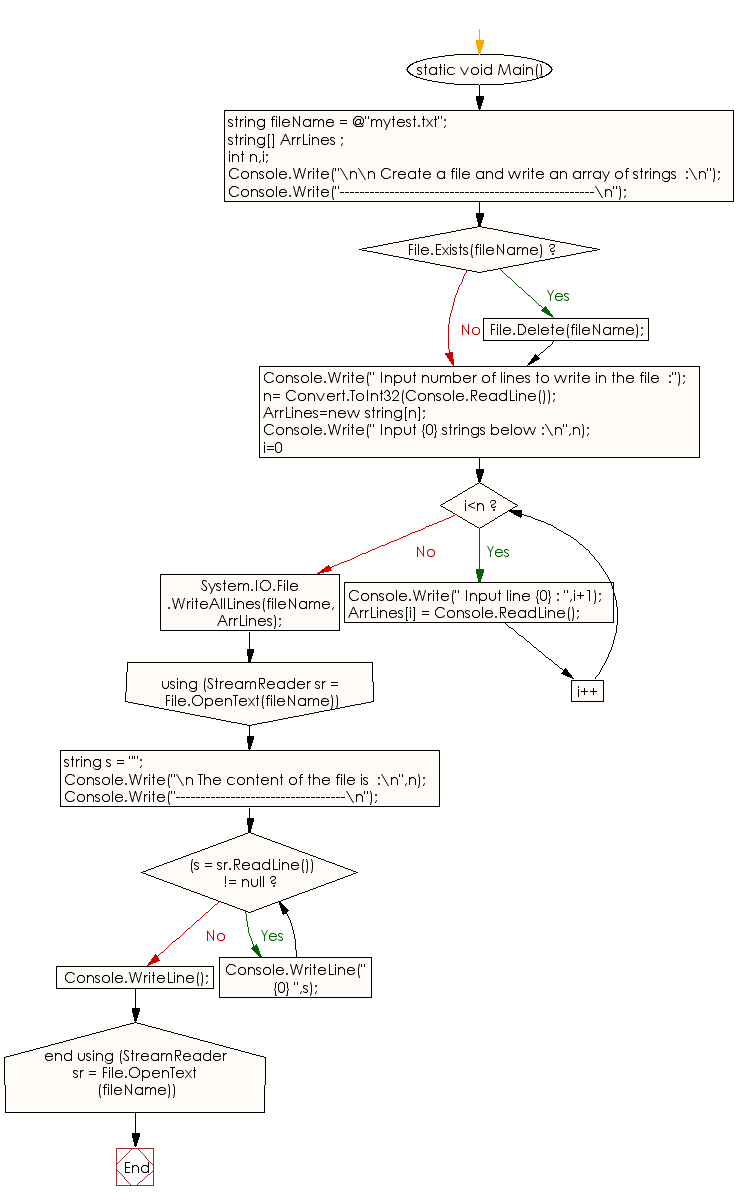
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a file with text and read the file.
Next: Write a program in C# Sharp to create and write some line of text into a file which does not contain a given string in a line.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.