C#: Display the pattern like a pyramid with an asterisk
Write a program in C# Sharp to make such a pattern like a pyramid with an asterisk.
The pattern is as follows:
* * * * * * * * * *
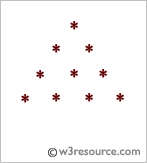
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise14 // Declaration of the Exercise14 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, spc, rows, k; // Declaration of variables 'i', 'j' for iteration, 'spc' for space count, 'rows' to store the number of rows, 'k' for iteration
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern like a pyramid with asterisk:\n"); // Displaying the purpose of the program
Console.Write("-------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of rows : "); // Prompting the user to input the number of rows
rows = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
spc = rows + 4 - 1; // Calculating the initial count of spaces for proper alignment
for (i = 1; i <= rows; i++) // Loop to iterate through each row
{
for (k = spc; k >= 1; k--) // Loop to print spaces before the asterisks for the pyramid pattern
{
Console.Write(" "); // Displaying spaces
}
for (j = 1; j <= i; j++) // Loop to print asterisks in each row 'i' times
{
Console.Write("* "); // Displaying an asterisk and a space
}
Console.Write("\n"); // Moving to the next line to form the pyramid pattern
spc--; // Decreasing the space count for the next row
}
}
}
Sample Output:
Display the pattern like pyramid with asterisk: ------------------------------------------------- Input number of rows : 6 * * * * * * * * * * * * * * * * * * * * *
Flowchart:
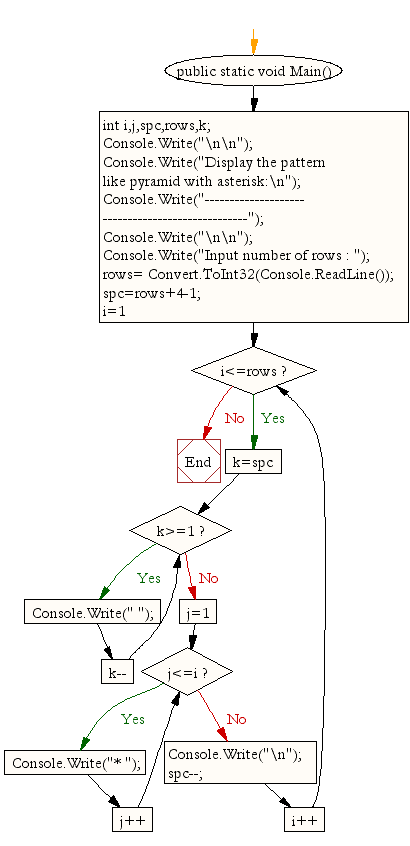
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to make such a pattern like a pyramid with numbers increased by 1
Next: Write a C# Sharp program to calculate the factorial of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.