C#: Check whether a number can be express as sum of two prime numbers
Write a program in C# Sharp to check whether a number can be expressed as the sum of two prime numbers.
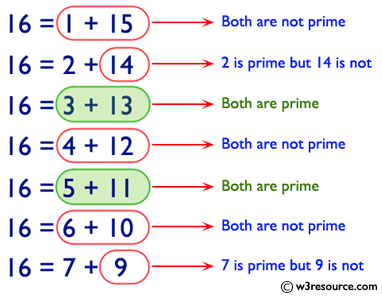
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise56 // Declaration of the Exercise56 class
{
public static void Main() // Main method, entry point of the program
{
int n, i, flg1 = 1, flg2 = 1, flg3 = 0, j; // Declaration of variables
Console.Write("\n\n");
Console.Write("Check whether a number can be expressed as the sum of two prime numbers:\n");
Console.Write("-------------------------------------------------------------------");
Console.Write("\n\n");
Console.Write("Input a positive integer: ");
n = Convert.ToInt32(Console.ReadLine()); // Taking user input for a positive integer
// Loop to find and display prime numbers that sum up to the given number 'n'
for (i = 3; i <= n / 2; i++)
{
// Check if 'i' is a prime number
flg1 = 1;
flg2 = 1;
// Loop to check if 'i' is prime
for (j = 2; j < i; j++)
{
if (i % j == 0)
{
flg1 = 0;
j = i;
}
}
// Loop to check if 'n-i' is prime
for (j = 2; j < n - i; j++)
{
if ((n - i) % j == 0)
{
flg2 = 0;
j = n - i;
}
}
// If 'i' and 'n-i' both are prime, display the combination
if (flg1 == 1 && flg2 == 1)
{
Console.Write("{0} = {1} + {2} \n", n, i, n - i);
flg3 = 1;
}
}
// If no combination is found, display appropriate message
if (flg3 == 0)
{
Console.Write("\n{0} can not be expressed as the sum of two prime numbers.\n\n", n);
}
}
}
Sample Output:
Check whether a number can be express as sum of two prime numbers: ------------------------------------------------------------------- Input a positive integer: 50 50 = 3 + 47 50 = 7 + 43 50 = 13 + 37 50 = 19 + 31
Flowchart:
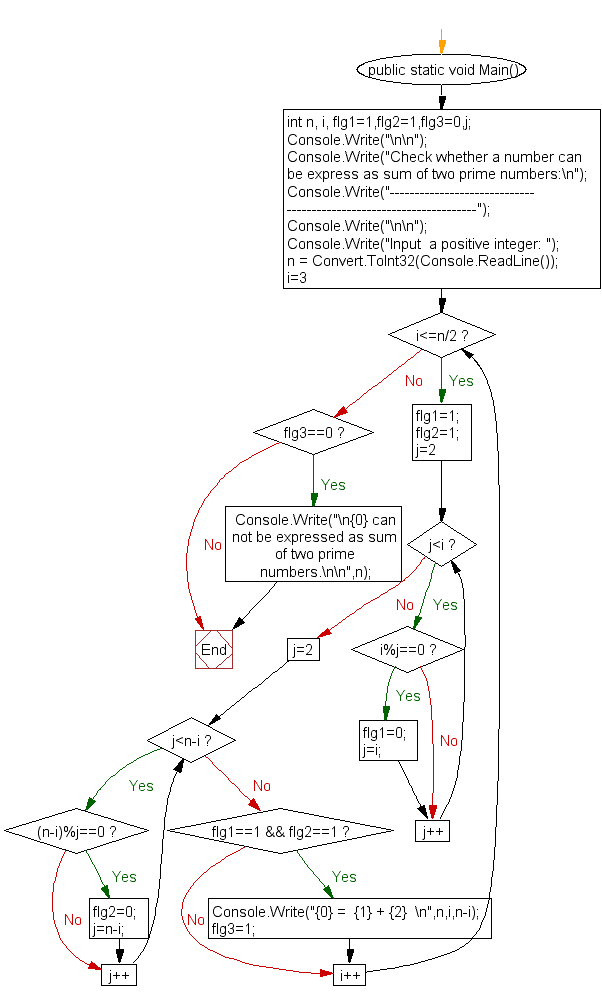
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to convert a decimal number to hexadecimal.
Next: Write a program in C# Sharp to print a string in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.