C#: Find the strings for a specific minimum length
Write a program in C# Sharp to find the strings for a specific minimum length.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
class LinqExercise22
{
static void Main(string[] args)
{
string[] arr1; // Declare an array to store strings
int n, i, ctr; // Declare variables to store the count, loop index, and minimum length
// Display a message indicating the purpose of the program
Console.Write("\nLINQ : Find the strings for a specific minimum length : ");
Console.Write("\n------------------------------------------------------\n");
// Ask user for the number of strings to store in the array
Console.Write("Input number of strings to store in the array :");
n = Convert.ToInt32(Console.ReadLine());
arr1 = new string[n]; // Initialize the array based on user input
// Prompt user to input strings into the array
Console.Write("\nInput {0} strings for the array :\n", n);
for (i = 0; i < n; i++)
{
Console.Write("Element[{0}] : ", i);
arr1[i] = Console.ReadLine(); // Store user input into the array
}
// Ask user for the minimum length of the item they want to find
Console.Write("\nInput the minimum length of the item you want to find : ");
ctr = Convert.ToInt32(Console.ReadLine());
// Use LINQ to find strings that meet the minimum length criteria
IEnumerable<string> objNew = from m in arr1
where m.Length >= ctr
orderby m
select m;
// Display the items that meet the minimum length criteria
Console.Write("\nThe items of minimum {0} characters are : \n", ctr);
foreach (string z in objNew)
Console.WriteLine("Item: {0}", z);
Console.ReadLine(); // Wait for user input before exiting
}
}
Sample Output:
LINQ : Find the strings for a specific minimum length : ------------------------------------------------------ Input number of strings to store in the array :3 Input 3 strings for the array : Element[0] : Welcome Element[1] : to Element[2] : W3resource Input the minimum length of the item you want to find : 10 The items of minimum 10 characters are : Item: W3resource
Visual Presentation:
Flowchart:
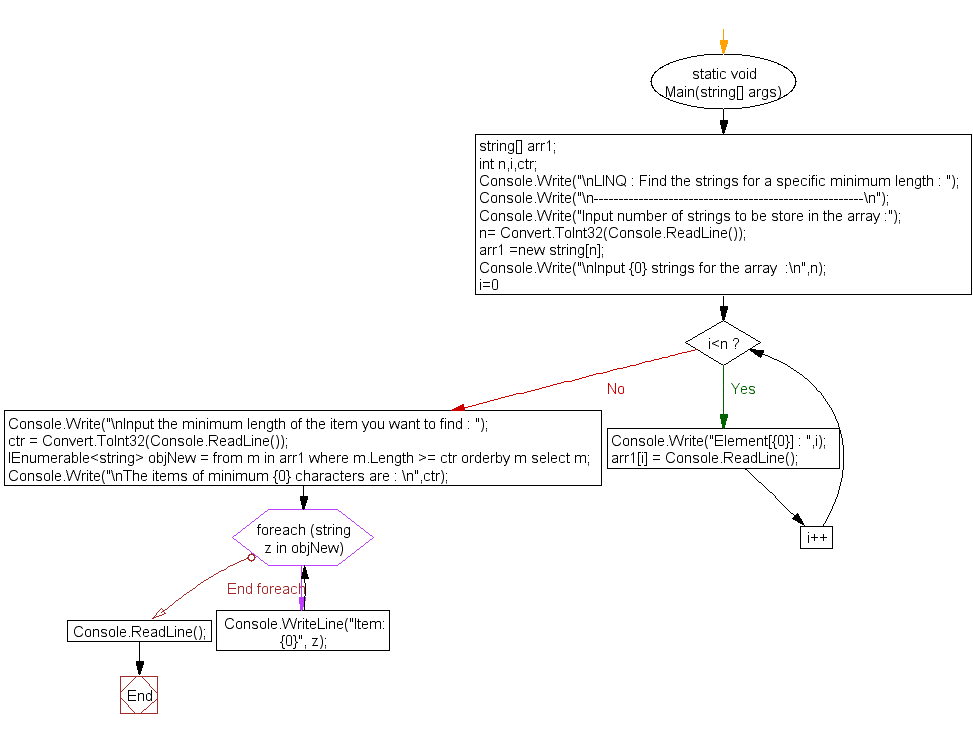
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to remove a range of items from a list by passing the start index and number of elements to remove.
Next: Write a program in C# Sharp to generate a Cartesian Product of two sets.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.