C#: When a structure and a class instance is passed to a method
Write a program in C# Sharp that shows what happens when a structure and a class instance are passed to a method.
Sample Solution:-
C# Sharp Code:
using System;
// Declaration of a class named newClass
class newClass
{
public int n; // Member variable 'n' of the class
}
// Declaration of a structure named newStruct
struct newStruct
{
public int n; // Member variable 'n' of the structure
}
class strucExer5
{
// Method to modify the value of 'n' in a newStruct instance
public static void trackStruct(newStruct st)
{
st.n = 8; // Modifying the 'n' value of the passed newStruct instance (won't affect original due to pass-by-value)
}
// Method to modify the value of 'n' in a newClass instance
public static void tracClass(newClass cl)
{
cl.n = 8; // Modifying the 'n' value of the passed newClass instance (will affect original due to pass-by-reference)
}
// Main method
public static void Main()
{
// Displaying a message indicating the scenario when a structure and a class instance are passed to a method
Console.Write("\n\nWhen a structure and a class instance is passed to a method :\n");
Console.Write("--------------------------------------------------------------\n");
// Creating instances of newStruct and newClass
newStruct ns = new newStruct();
newClass nc = new newClass();
// Assigning initial values to 'n' of newStruct and newClass instances
ns.n = 5;
nc.n = 5;
// Calling trackStruct method passing newStruct instance (will not modify original instance due to pass-by-value)
trackStruct(ns);
// Calling tracClass method passing newClass instance (will modify the original instance due to pass-by-reference)
tracClass(nc);
// Displaying the values of 'n' in both newStruct and newClass instances after method calls
Console.WriteLine("\nns.n = {0}", ns.n); // Displaying value of 'n' in newStruct instance (unchanged)
Console.WriteLine("nc.n = {0}\n", nc.n); // Displaying value of 'n' in newClass instance (modified)
}
}
Sample Output:
When a structure and a class instance is passed to a method : -------------------------------------------------------------- ns.n = 5 nc.n = 8
Flowchart:
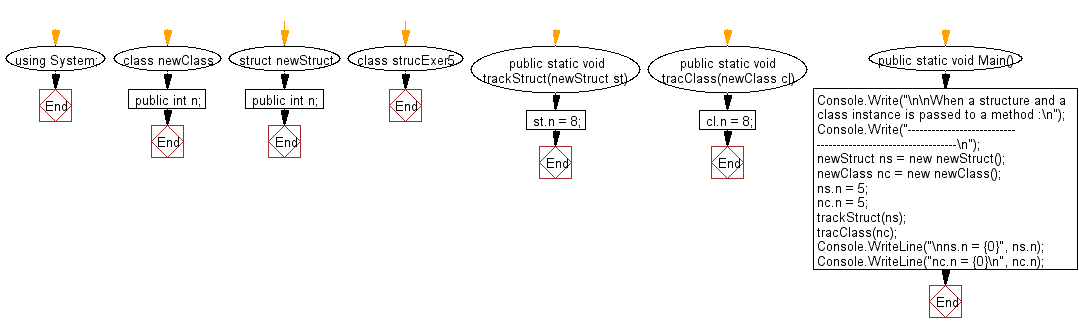
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to create a structure and assign the value and call it.
Next: Write a program in C# Sharp to declares a struct with a property, a method, and a private field.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.