JavaFX Application with multiple styled buttons
1. Style Multiple Buttons with Inline CSS
Write a JavaFX application with multiple buttons. Apply different styles to each button using inline styling and observe how various CSS properties impact their appearance.
Sample Solution:
JavaFx Code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
// Set the title for the primary stage
primaryStage.setTitle("Styled Buttons");
// Create Button 1 with specified styles
Button button1 = new Button("Button 1");
button1.setStyle("-fx-font-size: 14; -fx-background-color: #FF6347; -fx-text-fill: white;");
// Create Button 2 with specified styles
Button button2 = new Button("Button 2");
button2.setStyle("-fx-font-size: 16; -fx-background-color: #1E90FF; -fx-text-fill: white; -fx-border-color: black;");
// Create Button 3 with specified styles
Button button3 = new Button("Button 3");
button3.setStyle("-fx-font-size: 18; -fx-background-color: #32CD32; -fx-text-fill: white; -fx-border-radius: 5;");
// Create a VBox layout to hold the buttons with spacing
VBox vbox = new VBox(10, button1, button2, button3);
vbox.setSpacing(10);
// Create a scene with the VBox layout
Scene scene = new Scene(vbox, 300, 200);
// Set the scene on the primary stage
primaryStage.setScene(scene);
// Display the primary stage
primaryStage.show();
}
// Launch the application
public static void main(String[] args) {
launch(args);
}
}
Explanation:
In the exercise above,
- Button 1 has a red background, a smaller font size, and white text color.
- Button 2 has a blue background, a larger font size, white text color, and a black border.
- Button 3 has a green background, a larger font size, white text color, and rounded corners.
Run this JavaFX application to observe how different CSS properties applied using inline styling (setStyle) affect the buttons' appearance. Adjust the styles or properties as desired to see their impact on the button's appearance.
Sample Output:
Flowchart:
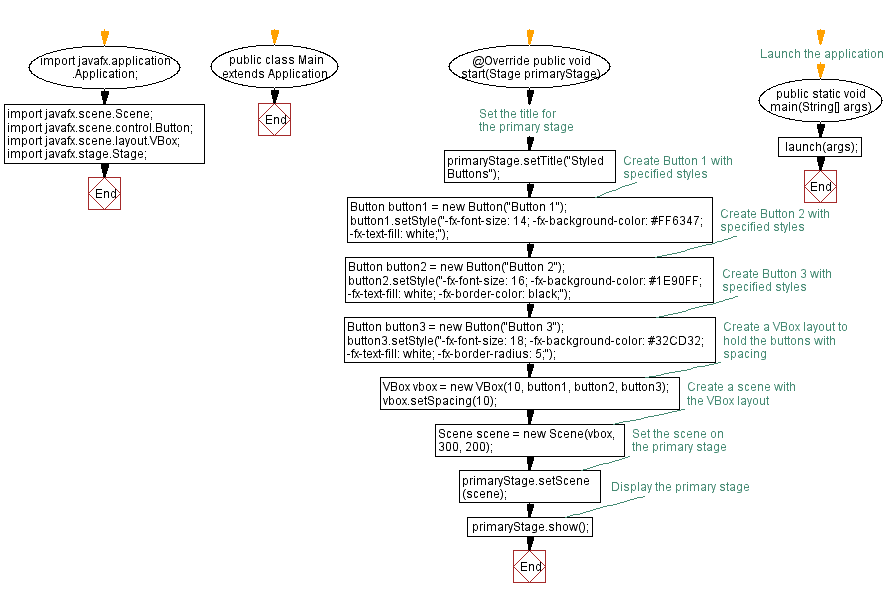
Go to:
PREV : JavaFx Styling and CSS Exercises Home.
NEXT : Style Form TextFields with CSS.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.