Java: Find the smallest number among three numbers
Find Smallest Number Among Three
Write a Java method to find the smallest number among three numbers.
Test Data:
Input the first number: 25
Input the Second number: 37
Input the third number: 29
Pictorial Presentation:
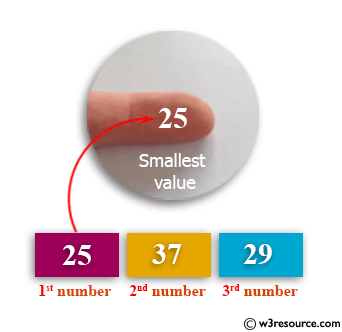
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise1 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input the first number: ");
double x = in.nextDouble();
System.out.print("Input the Second number: ");
double y = in.nextDouble();
System.out.print("Input the third number: ");
double z = in.nextDouble();
System.out.print("The smallest value is " + smallest(x, y, z)+"\n" );
}
public static double smallest(double x, double y, double z)
{
return Math.min(Math.min(x, y), z);
}
}
Sample Output:
Input the first number: 25 Input the Second number: 37 Input the third number: 29 The smallest value is 25.0
Flowchart:
For more Practice: Solve these Related Problems:
- Write a Java program to find the smallest number among three numbers using nested ternary operators.
- Write a Java program to determine the smallest number among three numbers passed as command-line arguments.
- Write a Java program to find the smallest number among three numbers without using any built‐in comparison methods.
- Write a Java program to extract three numbers from a comma-separated string and then find the smallest number.
Go to:
PREV : Java Methods Exercises Home.
NEXT : Compute Average of Three Numbers.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.