Java: Compute the average of three numbers
Compute Average of Three Numbers
Write a Java method to compute the average of three numbers.
Test Data:
Input the first number: 25
Input the second number: 45
Input the third number: 65
Pictorial Presentation:
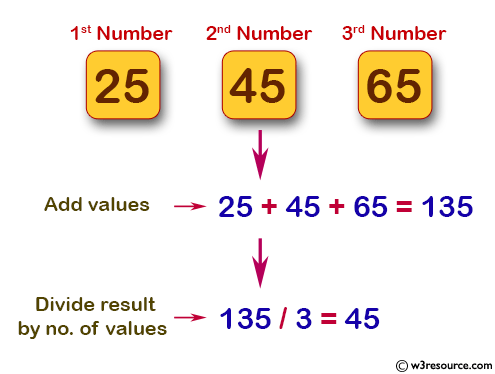
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise2 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input the first number: ");
double x = in.nextDouble();
System.out.print("Input the second number: ");
double y = in.nextDouble();
System.out.print("Input the third number: ");
double z = in.nextDouble();
System.out.print("The average value is " + average(x, y, z)+"\n" );
}
public static double average(double x, double y, double z)
{
return (x + y + z) / 3;
}
}
Sample Output:
Input the first number: 25 Input the second number: 45 Input the third number: 65 The average value is 45.0
Flowchart:
For more Practice: Solve these Related Problems:
- Write a Java program to compute the average of three floating-point numbers using recursion.
- Write a Java program to calculate the weighted average of three numbers with user-defined weights.
- Write a Java program to compute the average of three numbers provided in a single line input separated by commas.
- Write a Java program to compute the average of three numbers without directly using arithmetic operators.
Go to:
PREV : Find Smallest Number Among Three.
NEXT : Find Middle Character(s) of String.
Java Practice online:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.