Java String: indexOf() Method
indexOf() Method
Contents:
public int indexOf(int ch)
Returns the index within this string of the first occurrence of the specified character. If a character with value ch occurs in the character sequence represented by this String object, then the index (in Unicode code units) of the first such occurrence is returned. For values of ch in the range from 0 to 0xFFFF (inclusive), this is the smallest value k such that:
this.charAt(k) == ch
is true. For other values of ch, it is the smallest value k such that:
this.codePointAt(k) == ch
is true. In either case, if no such character occurs in this string, then -1 is returned.
Java Platform: Java SE 8
Syntax:
indexOf(int ch)
Parameters:
Name | Description | Type |
---|---|---|
ch | a character (Unicode code point). | int |
Return Value : the index of the first occurrence of the character in the character sequence represented by this object, or -1 if the character does not occur.
Return Value Type: int
Pictorial presentation of Java String indexOf() Method
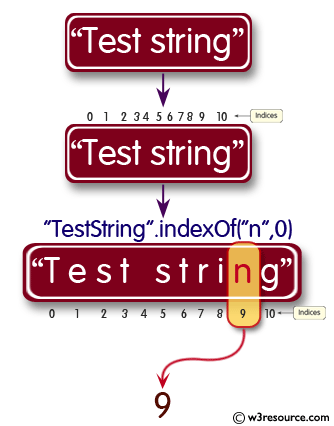
Example: Java String indexOf() Method
The following example shows the usage of java String() method.
public class Example {
public static void main(String[] args)
{
String str = "The quick brown fox jumps over the lazy dog.";
// Get the index of all the characters of the alphabet
// starting from the beginning of the String.
int a = str.indexOf("a", 0);
int b = str.indexOf("b", 0);
int c = str.indexOf("c", 0);
int d = str.indexOf("d", 0);
int e = str.indexOf("e", 0);
int f = str.indexOf("f", 0);
int g = str.indexOf("g", 0);
int h = str.indexOf("h", 0);
int i = str.indexOf("i", 0);
int j = str.indexOf("j", 0);
int k = str.indexOf("k", 0);
int l = str.indexOf("l", 0);
int m = str.indexOf("m", 0);
int n = str.indexOf("n", 0);
int o = str.indexOf("o", 0);
int p = str.indexOf("p", 0);
int q = str.indexOf("q", 0);
int r = str.indexOf("r", 0);
int s = str.indexOf("s", 0);
int t = str.indexOf("t", 0);
int u = str.indexOf("u", 0);
int v = str.indexOf("v", 0);
int w = str.indexOf("w", 0);
int x = str.indexOf("x", 0);
int y = str.indexOf("y", 0);
int z = str.indexOf("z", 0);
// Display the results of all the indexOf method calls.
System.out.println(" a b c d e f g h i j");
System.out.println("=========================");
System.out.println(a + " " + b + " " + c + " " + d + " " +
e + " " + f + " " + g + " " + h + " " +
i + " " + j + "\n");
System.out.println("k l m n o p q r s t");
System.out.println("===========================");
System.out.println(k + " " + l + " " + m + " " + n + " " +
o + " " + p + " " + q + " " + r + " " +
s + " " + t + "\n");
System.out.println("u v w x y z");
System.out.println("================");
System.out.println(u + " " + v + " " + w + " " + x + " " +
y + " " + z);
}
}
Output:
a b c d e f g h i j ========================= 36 10 7 40 2 16 42 1 6 20 k l m n o p q r s t =========================== 8 35 22 14 12 23 4 11 24 31 u v w x y z ================ 5 27 13 18 38 37
public int indexOf(int ch, int fromIndex)
Returns the index within this string of the first occurrence of the specified character, starting the search at the specified index.
If a character with value ch occurs in the character sequence represented by this String object at an index no smaller than fromIndex, then the index of the first such occurrence is returned. For values of ch in the range from 0 to 0xFFFF (inclusive), this is the smallest value k such that:
(this.charAt(k) == ch) && (k >= fromIndex)
is true. For other values of ch, it is the smallest value k such that:
is true. For other values of ch, it is the smallest value k such that:
(this.codePointAt(k) == ch) && (k >= fromIndex)
is true. In either case, if no such character occurs in this string at or after position fromIndex, then -1 is returned.
There is no restriction on the value of fromIndex. If it is negative, it has the same effect as if it were zero: this entire string may be searched. If it is greater than the length of this string, it has the same effect as if it were equal to the length of this string: -1 is returned.
All indices are specified in char values (Unicode code units).
Java Platform: Java SE 8
Syntax:
indexOf(int ch, int fromIndex)
Parameters:
Name | Description | Type |
---|---|---|
ch | a character (Unicode code point). | int |
fromIndex | the index to start the search from. | int |
Return Value: the index of the first occurrence of the character in the character sequence represented by this object that is greater than or equal to fromIndex, or -1 if the character does not occur.
Return Value Type: int
Example: Java String indexOf(int ch, int fromIndex) Method
The following example shows the usage of java String() method.
public class Example {
public static void main(String args[]) {
String str = "the quick brown fox jumps over the lazy dog.";
System.out.println();
System.out.println(str);
System.out.println("indexOf(t, 60) = " + str.indexOf('t', 30));
System.out.println();
}
}
Output:
the quick brown fox jumps over the lazy dog. indexOf(t, 60) = 31
public int indexOf(String str)
Returns the index within this string of the first occurrence of the specified substring.
The returned index is the smallest value k for which:
this.startsWith(str, k)
If no such value of k exists, then -1 is returned.
Java Platform: Java SE 8
Syntax:
indexOf(String str)
Parameters:
Name | Description | Type |
---|---|---|
str | the substring to search for. | int |
Return Value: the index of the first occurrence of the specified substring, or -1 if there is no such occurrence.
Return Value Type: int
Example: Java String indexOf(String, str) Method
The following example shows the usage of java String() method.
public class Example {
public static void main(String args[]) {
String str = "This is an example of indexOf from w3resource.com";
System.out.println();
System.out.println(str);
System.out.println("indexOf(w3r) = " + str.indexOf("w3r"));
System.out.println();
}
}
Output:
This is an example of indexOf from w3resource.com indexOf(w3r) = 35
public int indexOf(String str, int fromIndex)
Returns the index within this string of the first occurrence of the specified substring, starting at the specified index.
The returned index is the smallest value k for which:
k >= fromIndex && this.startsWith(str, k)
If no such value of k exists, then -1 is returned.
Java Platform: Java SE 8
Syntax:
indexOf(String str, int fromIndex)
Parameters:
Name | Description | Type |
---|---|---|
str | the substring to search for. | int |
fromIndex | the index from which to start the search. | int |
Return Value: the index of the first occurrence of the specified substring, starting at the specified index, or -1 if there is no such occurrence.
Return Value Type: int
Example: Java String indexOf(String str, int fromIndex) Method
The following example shows the usage of java String() method.
public class Example {
public static void main(String args[]) {
String str = "the quick brown fox jumps over the lazy dog.";
System.out.println();
System.out.println(str);
System.out.println("indexOf(the, 40) = " + str.indexOf("the", 30));
System.out.println();
}
}
Output:
the quick brown fox jumps over the lazy dog. indexOf(the, 40) = 31
Java Code Editor:
Previous:hashCode Method
Next:intern Method