JavaScript: Check from three given numbers that two or all of them have the same rightmost digit
JavaScript Basic: Exercise-43 with Solution
Check Rightmost Digits of Three Numbers
Write a JavaScript program to check from three given numbers (non negative integers) that two or all of them have the same rightmost digit.
This JavaScript program checks whether from three given non-negative integers, two or all of them have the same rightmost digit. It examines the last digit of each number and compares them to determine if they match, returning true if there's a match and false otherwise.
Visual Presentation:
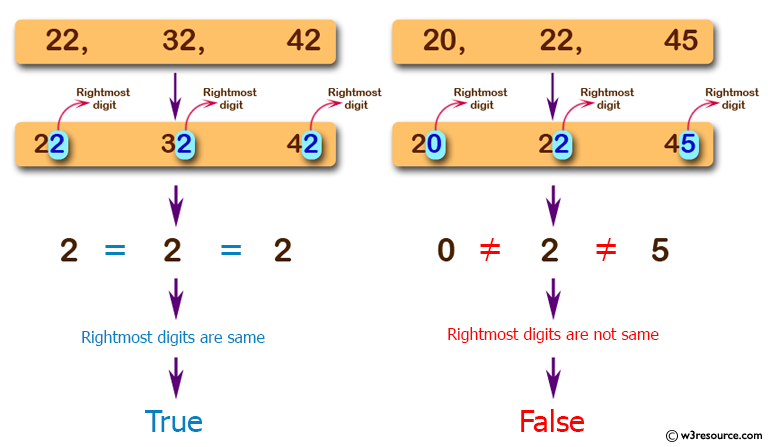
Sample Solution:
JavaScript Code:
// Define a function named same_last_digit with parameters p, q, and r
function same_last_digit(p, q, r) {
// Return true if the last digit of p is equal to the last digit of q or r,
// or if the last digit of q is equal to the last digit of r
return (p % 10 === q % 10) ||
(p % 10 === r % 10) ||
(q % 10 === r % 10);
}
// Log the result of calling same_last_digit with the arguments 22, 32, and 42 to the console
console.log(same_last_digit(22, 32, 42));
// Log the result of calling same_last_digit with the arguments 102, 302, and 2 to the console
console.log(same_last_digit(102, 302, 2));
// Log the result of calling same_last_digit with the arguments 20, 22, and 45 to the console
console.log(same_last_digit(20, 22, 45));
Output:
true true false
Live Demo:
See the Pen JavaScript: Check from three given numbers - basic-ex-43 by w3resource (@w3resource) on CodePen.
Flowchart:
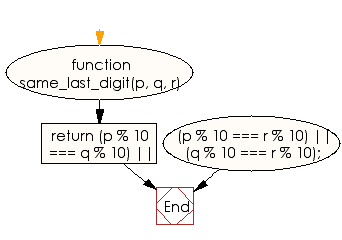
ES6 Version:
// Define a function named same_last_digit using arrow function syntax with parameters p, q, and r
const same_last_digit = (p, q, r) => {
// Check if the last digit of p is equal to the last digit of q or r
// Check if the last digit of q is equal to the last digit of r
return (p % 10 === q % 10) || (p % 10 === r % 10) || (q % 10 === r % 10);
};
// Log the result of calling same_last_digit with the arguments 22, 32, and 42 to the console
console.log(same_last_digit(22, 32, 42));
// Log the result of calling same_last_digit with the arguments 102, 302, and 2 to the console
console.log(same_last_digit(102, 302, 2));
// Log the result of calling same_last_digit with the arguments 20, 22, and 45 to the console
console.log(same_last_digit(20, 22, 45));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if at least two out of three numbers share the same rightmost digit using arithmetic operations.
- Write a JavaScript program that extracts the last digit of three numbers and compares them, returning true if at least two match.
- Write a JavaScript program that determines if two or more of three numbers have identical last digits without converting them to strings.
Go to:
PREV : Check Numbers in Strict or Soft Increasing Mode.
NEXT : Evaluate if Integer is ≥20 and Less Than Another.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.