JavaScript: Check two given integer values and return true if one of the number is 15 or if their sum or difference is 15
JavaScript Basic: Exercise-45 with Solution
Check if Integer is 15, or Sum/Difference is 15
Write a JavaScript program that checks two integer values and returns true if either one is 15 or if their sum or difference is 15.
This JavaScript program evaluates two integer values to determine if either one is equal to 15 or if their sum or difference equals 15. If any of these conditions are met, it returns true; otherwise, it returns false.
Visual Presentation:
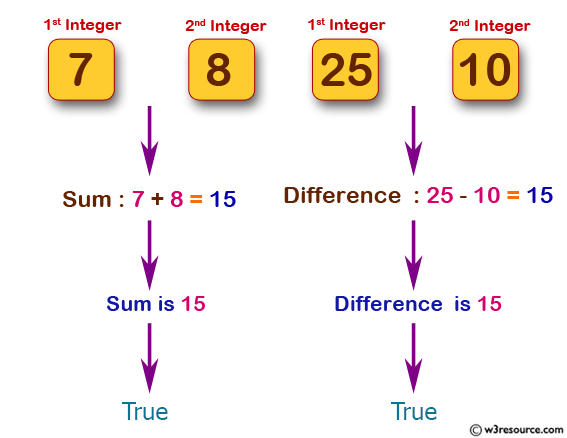
Sample Solution:
JavaScript Code:
// Define a function named test_number with parameters x and y
function test_number(x, y) {
// Return true if x is equal to 15, y is equal to 15, the sum of x and y is equal to 15,
// or the absolute difference between x and y is equal to 15; otherwise, return false.
return (x === 15 || y === 15 || x + y === 15 || Math.abs(x - y) === 15);
}
// Log the result of calling test_number with the arguments 15 and 9 to the console
console.log(test_number(15, 9));
// Log the result of calling test_number with the arguments 25 and 15 to the console
console.log(test_number(25, 15));
// Log the result of calling test_number with the arguments 7 and 8 to the console
console.log(test_number(7, 8));
// Log the result of calling test_number with the arguments 25 and 10 to the console
console.log(test_number(25, 10));
// Log the result of calling test_number with the arguments 5 and 9 to the console
console.log(test_number(5, 9));
// Log the result of calling test_number with the arguments 7 and 9 to the console
console.log(test_number(7, 9));
// Log the result of calling test_number with the arguments 9 and 25 to the console
console.log(test_number(9, 25));
Output:
true true true true false false false
Live Demo:
See the Pen JavaScript: Check two given integer values and return true if one of the number is 15 or if their sum or difference is 15 - basic-ex-45 by w3resource (@w3resource) on CodePen.
Flowchart:
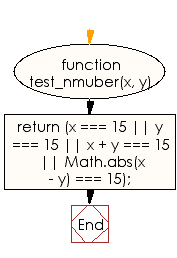
ES6 Version:
// Define a function named test_number with parameters x and y
function test_number(x, y) {
// Return true if x is equal to 15, y is equal to 15, the sum of x and y is equal to 15, or the absolute difference between x and y is equal to 15
return (x === 15 || y === 15 || x + y === 15 || Math.abs(x - y) === 15);
}
// Log the result of calling test_number with the arguments 15 and 9 to the console
console.log(test_number(15, 9));
// Log the result of calling test_number with the arguments 25 and 15 to the console
console.log(test_number(25, 15));
// Log the result of calling test_number with the arguments 7 and 8 to the console
console.log(test_number(7, 8));
// Log the result of calling test_number with the arguments 25 and 10 to the console
console.log(test_number(25, 10));
// Log the result of calling test_number with the arguments 5 and 9 to the console
console.log(test_number(5, 9));
// Log the result of calling test_number with the arguments 7 and 9 to the console
console.log(test_number(7, 9));
// Log the result of calling test_number with the arguments 9 and 25 to the console
console.log(test_number(9, 25));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if either of two integers is 15 or if their sum or difference equals 15.
- Write a JavaScript program that verifies if one of two numbers is 15 or if combining them by addition or subtraction results in 15.
- Write a JavaScript program that takes two integers and determines if one is 15 or if their sum/difference is 15, returning a boolean.
Go to:
PREV : Evaluate if Integer is ≥20 and Less Than Another.
NEXT : Check if Only One Integer is Multiple of 7 or 11.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.