JavaScript: Capitalize the first letter of each word of a given string
JavaScript Basic: Exercise-50 with Solution
Capitalize First Letter of Each Word in String
Write a JavaScript program to capitalize the first letter of each word in a given string.
This JavaScript program capitalizes the first letter of each word in a given string. It splits the string into words, capitalizes the first letter of each word, and then joins the words back into a single string.
Visual Presentation:
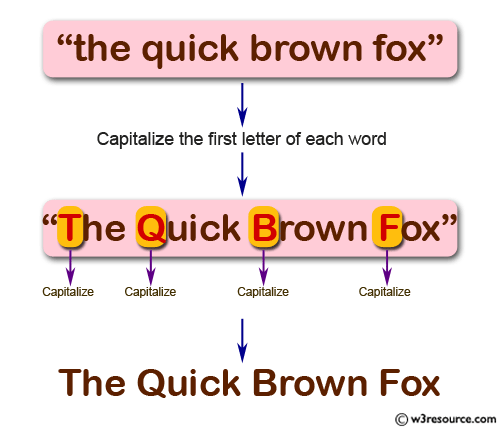
Sample Solution:
JavaScript Code:
// Define a function named capital_letter with parameter str
function capital_letter(str)
{
// Split the input string into an array of words
str = str.split(" ");
// Iterate through each word in the array
for (var i = 0, x = str.length; i < x; i++) {
// Capitalize the first letter of each word and concatenate it with the rest of the word
str[i] = str[i][0].toUpperCase() + str[i].substr(1);
}
// Join the modified array of words back into a string
return str.join(" ");
}
// Log the result of calling capital_letter with the given string to the console
console.log(capital_letter("Write a JavaScript program to capitalize the first letter of each word of a given string."));
Output:
Write A JavaScript Program To Capitalize The First Letter Of Each Word Of A Given String.
Live Demo:
See the Pen JavaScript - capitalize the first letter of each word of a given string - basic-ex-50 by w3resource (@w3resource) on CodePen.
Flowchart:
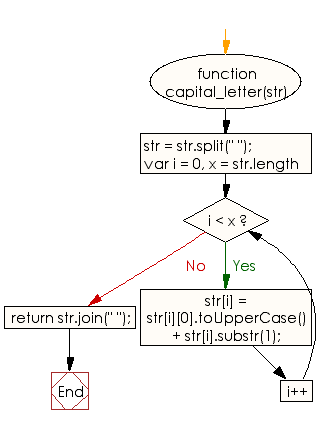
ES6 Version:
// Define a function named capital_letter with parameter str
const capital_letter = (str) => {
// Split the input string into an array of words
str = str.split(" ");
// Iterate through each word in the array
for (let i = 0, x = str.length; i < x; i++) {
// Capitalize the first letter of each word and concatenate it with the rest of the word
str[i] = str[i][0].toUpperCase() + str[i].substr(1);
}
// Join the modified array of words back into a string
return str.join(" ");
};
// Log the result of calling capital_letter with the given string to the console
console.log(capital_letter("Write a JavaScript program to capitalize the first letter of each word of a given string."));
The following tool visualize what the computer is doing step-by-step as it executes the said program:
-->
For more Practice: Solve these Related Problems:
- Write a JavaScript program that capitalizes the first letter of each word in a string and lowercases the remaining letters.
- Write a JavaScript program that transforms a sentence so that each word's first letter is capitalized and then reverses the entire word order.
- Write a JavaScript program that converts the first letter of every word in a string to uppercase, ignoring words that are shorter than three letters.
Go to:
PREV : Replace Each Character with Next Alphabet Letter.
NEXT : Transform Number to Hours and Minutes.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.