JavaScript: Reverse the elements of a given array of integers length 3
JavaScript Basic: Exercise-73 with Solution
Reverse Elements of Array (Length 3)
Write a JavaScript program to reverse the elements of a given array of integers of length 3.
The program reverses the order of elements in an array of integers of length 3. It swaps the first and last elements, resulting in the array being in reverse order from its original state.
Visual Presentation:
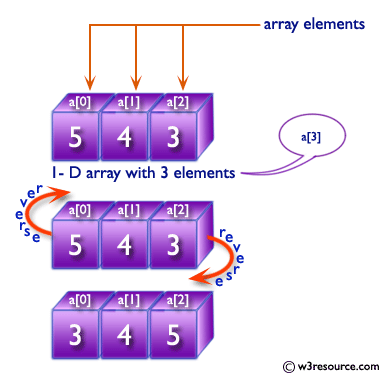
Sample Solution:
JavaScript Code:
// Define a function named reverse3 that takes an array as a parameter
function reverse3(array) {
// Use the map method to iterate over the array and reverse the order
return array.map((element, idx, arr) => arr[(arr.length - 1) - idx]);
}
// Call the function with sample arguments and log the results to the console
console.log(reverse3([5, 4, 3])); // Output: [3, 4, 5]
console.log(reverse3([1, 0, -1])); // Output: [-1, 0, 1]
console.log(reverse3([2, 3, 1])); // Output: [1, 3, 2]
Output:
[3,4,5] [-1,0,1] [1,3,2]
Live Demo:
See the Pen JavaScript - Reverse the elements of a given array of integers length 3 - basic-ex-73 by w3resource (@w3resource) on CodePen.
Flowchart:
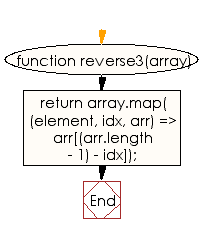
ES6 Version:
// Define a function named reverse3 that takes an array as a parameter
const reverse3 = (array) => array.map((element, idx, arr) => arr[(arr.length - 1) - idx]);
// Call the function with sample arguments and log the results to the console
console.log(reverse3([5, 4, 3])); // Output: [3, 4, 5]
console.log(reverse3([1, 0, -1])); // Output: [-1, 0, 1]
console.log(reverse3([2, 3, 1])); // Output: [1, 3, 2]
For more Practice: Solve these Related Problems:
- Write a JavaScript program that reverses the order of elements in a three-element array without using the built-in reverse() method.
- Write a JavaScript function that swaps the first and third elements of a length-3 array to achieve a reversed order.
- Write a JavaScript program that takes an array of three numbers and outputs a new array with the elements in reverse order using manual iteration.
Go to:
PREV : Check if First and Last Elements Are Same.
NEXT : Set All Elements to Largest of First/Last in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.