JavaScript: Check whether the first and last elements are equal of a given array of integers length 3
JavaScript Basic: Exercise-72 with Solution
Check if First and Last Elements Are Same
Write a JavaScript program to check whether the first and last elements are the same in a given array of integers of length 3.
The program checks if the first and last elements in an array of integers of length 3 are the same. If they are identical, it returns true; otherwise, it returns false.
Visual Presentation:
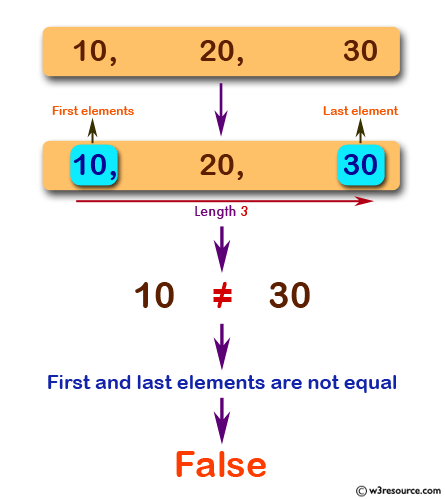
Sample Solution:
JavaScript Code:
// Define a function named first_last_same with a parameter nums
function first_last_same(nums) {
// Calculate the index of the last element in the array
var end = nums.length - 1;
// Check if the array has at least one element
if (nums.length >= 1) {
// Return true if the first and last elements of the array are equal, otherwise return false
return nums[0] == nums[end];
} else {
// Return false if the array is empty
return false;
}
}
// Call the function with sample arguments and log the results to the console
console.log(first_last_same([10, 20, 30]));
console.log(first_last_same([10, 20, 30, 10]));
console.log(first_last_same([20, 20, 20]));
Output:
false true true
Live Demo:
See the Pen JavaScript - check whether the first and last elements are equal of a given array of integers length 3 - basic-ex-72 by w3resource (@w3resource) on CodePen.
Flowchart:
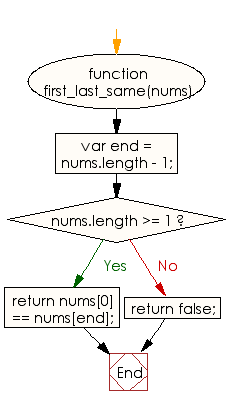
ES6 Version:
// Define a function named first_last_same with a parameter nums using arrow function syntax
const first_last_same = nums => {
// Store the last index of the array
const end = nums.length - 1;
// Check if the array has at least one element
if (nums.length >= 1) {
// Use the equality operator (==) to compare the first and last elements
return nums[0] === nums[end];
} else {
// Return false if the array is empty
return false;
}
};
// Call the function with sample arguments and log the results to the console
console.log(first_last_same([10, 20, 30]));
console.log(first_last_same([10, 20, 30, 10]));
console.log(first_last_same([20, 20, 20]));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether 1 appears in first or last position of a given array of integers.
Next: JavaScript program to reverse the elements of a given array of integers length 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-basic-exercise-72.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics