JavaScript: Least common multiple (LCM) of two numbers
JavaScript Math: Exercise-10 with Solution
LCM of Two Numbers
Write a JavaScript function to get the least common multiple (LCM) of two numbers.
Note :
According to Wikipedia - A common multiple is a number that is a multiple of two or more integers. The common multiples of 3 and 4 are 0, 12, 24, .... The least common multiple (LCM) of two numbers is the smallest number (not zero) that is a multiple of both.
Test Data:
console.log(lcm_two_numbers(3,15));
console.log(lcm_two_numbers(10,15));
Output :
15
30
Explanation of L.C.M.:
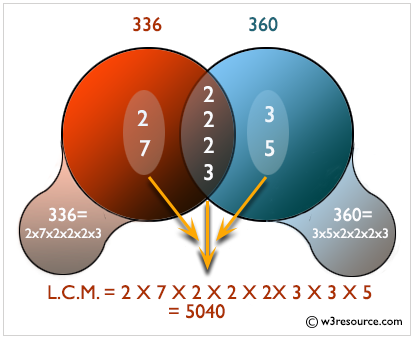
Visual Presentation of L.C.M. of two numbers:
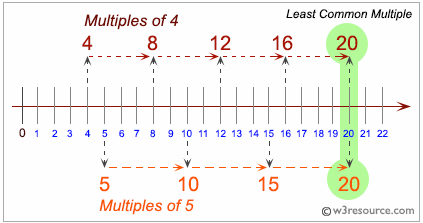
Sample Solution:
JavaScript Code:
// Define a function named lcm_two_numbers that calculates the least common multiple (LCM) of two numbers.
function lcm_two_numbers(x, y) {
// Check if both x and y are of type number, if not, return false.
if ((typeof x !== 'number') || (typeof y !== 'number'))
return false;
// Calculate the LCM using the formula: LCM(x, y) = |x * y| / GCD(x, y).
return (!x || !y) ? 0 : Math.abs((x * y) / gcd_two_numbers(x, y));
}
// Define a function named gcd_two_numbers that calculates the greatest common divisor (GCD) of two numbers.
function gcd_two_numbers(x, y) {
// Take the absolute values of x and y to ensure positivity.
x = Math.abs(x);
y = Math.abs(y);
// Use the Euclidean algorithm to find the GCD.
while(y) {
var t = y;
y = x % y;
x = t;
}
// Return the GCD, which is stored in x after the loop.
return x;
}
// Output the LCM of 3 and 15 to the console.
console.log(lcm_two_numbers(3, 15));
// Output the LCM of 10 and 15 to the console.
console.log(lcm_two_numbers(10, 15));
Output:
15 30
Flowchart:
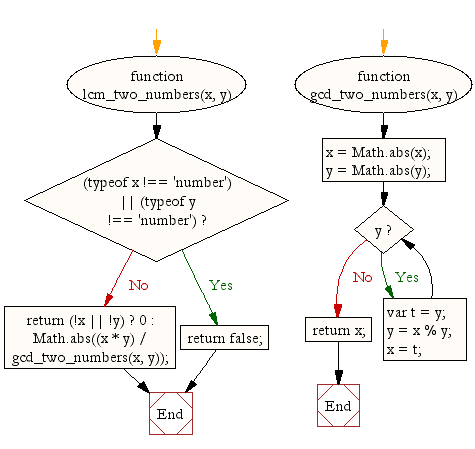
Live Demo:
See the Pen javascript-math-exercise-10 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the LCM of two numbers using their GCD.
- Write a JavaScript function that computes the LCM iteratively without recursion.
- Write a JavaScript function that calculates the LCM by first decomposing numbers into their prime factors.
- Write a JavaScript function that finds the LCM of two numbers and handles edge cases such as inputs of zero.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find the GCD (greatest common divisor) of more than 2 integers.
Next: Write a JavaScript function to get the least common multiple (LCM) of more than 2 integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.