JavaScript: Parse an URL
JavaScript Object: Exercise-12 with Solution
Parse URL
Write a JavaScript function to parse an URL.
Sample Solution: -
JavaScript Code:
function parse_URL(url) {
var a = document.createElement('a');
a.href = url;
return {
source: url,
protocol: a.protocol.replace(':', ''),
host: a.hostname,
port: a.port,
query: a.search,
params: (function () {
var ret = {},
seg = a.search.replace(/^\?/, '').split('&'),
len = seg.length,
i = 0,
s;
for (; i < len; i++) {
if (!seg[i]) {
continue;
}
s = seg[i].split('=');
ret[s[0]] = s[1];
}
return ret;
})(),
file: (a.pathname.match(/\/([^\/?#]+)$/i) || [, ''])[1],
hash: a.hash.replace('#', ''),
path: a.pathname.replace(/^([^\/])/, '/$1'),
relative: (a.href.match(/tps?:\/\/[^\/]+(.+)/) || [, ''])[1],
segments: a.pathname.replace(/^\//, '').split('/')
};
}
console.log(parse_URL('https://github.com/pubnub/python/search?utf8=%E2%9C%93&q=python'));
Output:
{"source":"https://github.com/pubnub/python/search?utf8=%E2%9C%93&q=python","protocol":"https","host":"github.com","port":"","query":"?utf8=%E2%9C%93&q=python","params":{"utf8":"%E2%9C%93","q":"python"},"file":"search","hash":"","path":"/pubnub/python/search","relative":"/pubnub/python/search?utf8=%E2%9C%93&q=python","segments":["pubnub","python","search"]}
Flowchart:
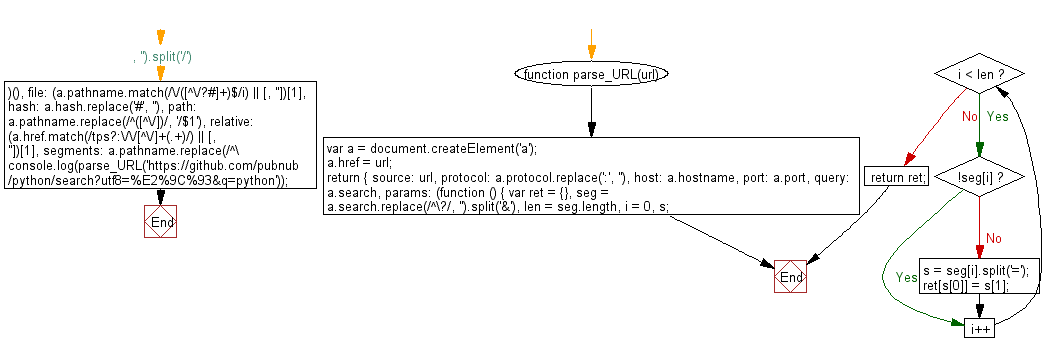
Live Demo:
See the Pen javascript-object-exercise-12 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that splits a URL into its components (protocol, host, port, pathname, query, hash) and returns an object.
- Write a JavaScript function that validates a URL string before parsing and returns an error if invalid.
- Write a JavaScript function that extracts the query parameters from a URL and returns them as key-value pairs.
- Write a JavaScript function that handles URLs with missing components and fills in default values for them.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to print all the methods in an JavaScript object.
Next: Write a JavaScript function to retrieve all the names of object's own and inherited properties.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.