JavaScript: Truncate a string to a certain number of words
JavaScript String: Exercise-24 with Solution
Truncate by Words
Write a JavaScript function to truncate a string to a certain number of words.
Test Data:
console.log(truncate('The quick brown fox jumps over the lazy dog', 4));
Output:
"The quick brown fox"
Visual Presentation:
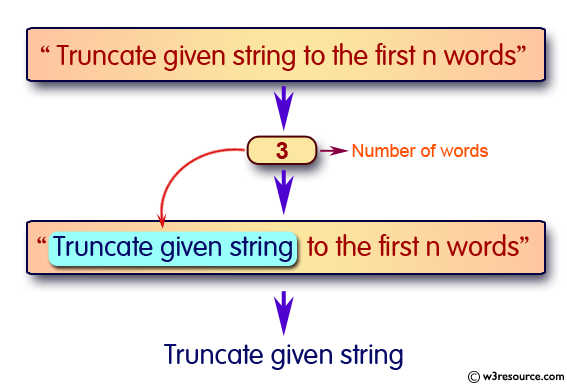
Sample Solution:
JavaScript Code:
// Define a function named truncate that takes two parameters: a string (str) and the number of words to truncate to (no_words)
function truncate(str, no_words) {
// Split the input string into an array of words using the space character (" ") as the delimiter, then extract a portion of the array containing the specified number of words using the splice method, and finally join the selected words back into a single string with spaces between them
return str.split(" ").splice(0,no_words).join(" ");
}
// Call the truncate function with a sample string and a specified number of words to truncate to, then output the result
console.log(truncate('The quick brown fox jumps over the lazy dog', 4));
Output:
The quick brown fox
Explanation:
In the exercise above,
The JavaScript code defines a function called "truncate()" that takes two parameters: a string ('str') and the number of words to truncate to ('no_words'). Inside the function:
- The input string is split into an array of words using the space character (" ") as the delimiter.
- Then, the "splice()" method is used to extract a portion of the array starting from the beginning and containing the specified number of words ('no_words').
- Finally, the selected words are joined back into a single string with spaces between them.
Flowchart:
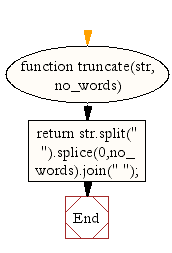
Live Demo:
See the Pen JavaScript Truncate a string to a certain number of words - string-ex-24 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that truncates a string to a specified number of words without breaking any word.
- Write a JavaScript function that splits a string into words and then rejoins only the first n words.
- Write a JavaScript function that validates the word count and returns the original string if it has fewer words than specified.
- Write a JavaScript function that handles punctuation correctly when truncating by words.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to strip leading and trailing spaces from a string.
Next: Write a JavaScript function to alphabetize a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.