PHP function to check file existence by path
2. Check if the file exists
Write a PHP function that takes a file path as input and checks if the file exists.
Sample Solution:
PHP Code :
<?php
function checkFileExists($filePath) {
if (file_exists($filePath)) {
echo "File exists at path: " . $filePath;
} else {
echo "File does not exist at path: " . $filePath;
}
}
// Usage:
$filePath = "test.txt";
//$filePath = "i:/test.txt";
checkFileExists($filePath);
?>
Sample Output:
File does not exist at path: test.txt File exists at path: i:/test.txt
Explanation:
In the above exercise -
- Inside the function, we use the file_exists() function to check if the file exists at the given path. If the file exists, we display a message indicating its existence along with the file path. If the file does not exist, we display a message indicating that the file does not exist along with the file path.
- To use this function, you can provide the file path as an argument when calling the checkFileExists() function.
- When you run this program, it will output either "File exists at path: <file path>" or "File does not exist at path: <file path>", depending on whether the file exists or not.
Flowchart:
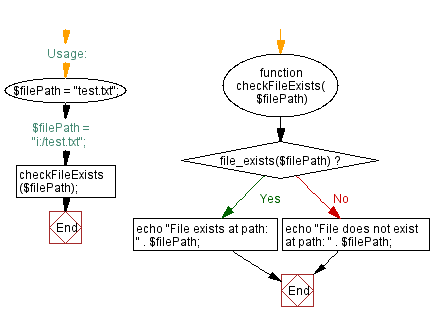
For more Practice: Solve these Related Problems:
- Write a PHP function that checks for multiple file paths and returns an array of files that exist.
- Write a PHP script to check if a file exists and is accessible by a specific user using permissions.
- Write a PHP function to recursively check if a file exists in nested directories.
- Write a PHP script to verify if a file exists and validate its MIME type before displaying its contents.
Go to:
PREV : Read and display contents of a text file.
NEXT : Count the number of lines in a text file.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.