PHP Array Exercises : Set union of two arrays
48. Set Union of Two Arrays
Write a PHP function to set union of two arrays.
Sample Solution:
PHP Code:
<?php
// Function to calculate the union of two arrays
function array_union($x, $y)
{
// Use array_merge to combine three arrays:
// 1. Intersection of $x and $y
// 2. Elements in $x that are not in $y
// 3. Elements in $y that are not in $x
$aunion = array_merge(
array_intersect($x, $y), // Intersection of $x and $y
array_diff($x, $y), // Elements in $x but not in $y
array_diff($y, $x) // Elements in $y but not in $x
);
// Return the resulting array representing the union
return $aunion;
}
// Example arrays
$a = array(1, 2, 3, 4);
$b = array(2, 3, 4, 5, 6);
// Call the function and display the result
print_r(array_union($a, $b));
?>
Output:
Array ( [0] => 2 [1] => 3 [2] => 4 [3] => 1 [4] => 5 [5] => 6 )
Flowchart:
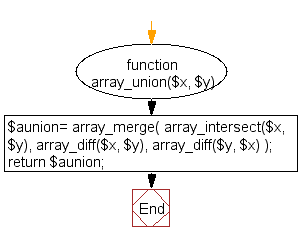
For more Practice: Solve these Related Problems:
- Write a PHP function to compute the union of two arrays and return the resulting array containing all unique values.
- Write a PHP script to merge two arrays, remove duplicates, and display the final union array.
- Write a PHP program to calculate the union of arrays without using array_merge() and array_unique(), instead using loops.
- Write a PHP script to combine two arrays and then sort the union while preserving unique elements only.
Go to:
PREV : Get an Array with First Key and Value.
NEXT : Get Array Entries with Specific Keys.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.