PHP Date Exercises : Check whether a date is a weekend or not
15. Check if Date is a Weekend
Write a PHP script to check whether a date is a weekend or not.
Sample Solution:
PHP Code:
<?php
$dt='2011-01-04'; // Assigning the date '2011-01-04' to the variable $dt.
$dt1 = strtotime($dt); // Converting the date string to a Unix timestamp and storing it in $dt1.
$dt2 = date("l", $dt1); // Formatting the Unix timestamp $dt1 to retrieve the day of the week in full textual representation (e.g., 'Monday') and storing it in $dt2.
$dt3 = strtolower($dt2); // Converting the day of the week to lowercase and storing it in $dt3.
if (($dt3 == "saturday") || ($dt3 == "sunday")) { // Checking if the day is Saturday or Sunday.
echo $dt3.' is weekend'."\n"; // Outputting that it's a weekend if the day is Saturday or Sunday.
} else {
echo $dt3.' is not weekend'."\n"; // Outputting that it's not a weekend if the day is not Saturday or Sunday.
}
?>
Output:
tuesday is not weekend
Explanation:
In the exercise above,
- $dt='2011-01-04';: Assigning the date '2011-01-04' to the variable '$dt'.
- $dt1 = strtotime($dt);: Converting the date string stored in '$dt' to a Unix timestamp and storing it in the variable '$dt1'.
- $dt2 = date("l", $dt1);: Formatting the Unix timestamp stored in '$dt1' to retrieve the day of the week in full textual representation (e.g., 'Monday') and storing it in the variable '$dt2'.
- $dt3 = strtolower($dt2);: Converting the day of the week stored in '$dt2' to lowercase and storing it in the variable '$dt3'.
- Checking if the day stored in '$dt3' is Saturday or Sunday using an if-else statement.
- Outputting whether the day is a weekend day or not based on the result of the if-else condition.
Flowchart :
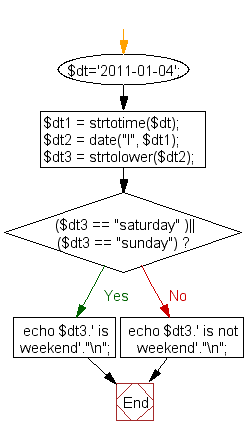
For more Practice: Solve these Related Problems:
- Write a PHP script to check if a given date falls on a weekend (Saturday or Sunday) and return a boolean.
- Write a PHP function that accepts a date string and outputs a message indicating whether the date is a weekend or a weekday.
- Write a PHP program to iterate over a range of dates and list only those that fall on weekends.
- Write a PHP script to use the date() function to check if today is a weekend and display a corresponding special message.
Go to:
PREV : Current Date/Time for Australia/Melbourne.
NEXT : Date Arithmetic: Add/Subtract Days.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.