PHP for loop Exercises: Print alphabet pattern Z
38. Alphabet Pattern 'Z'
Write a PHP program to print alphabet pattern Z.
Visual Presentation:
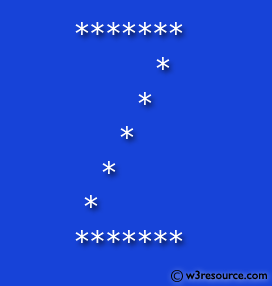
Sample Solution:
PHP Code:
<?php
// Loop for rows
for ($row=0; $row<7; $row++)
{
// Loop for columns
for ($column=0; $column<=7; $column++)
{
// Condition to determine whether to print '*' or ' '
if ((($row == 0 or $row == 6) and $column >= 0 and $column <= 6) or $row+$column==6)
echo "*"; // Print '*' if condition is met
else
echo " "; // Print ' ' if condition is not met
}
echo "\n"; // Move to the next line after each row is printed
}
?>
Output:
******* * * * * * *******
Explanation:
In the exercise above,
- The code starts with a PHP opening tag <?php.
- It utilizes a nested loop structure to iterate over rows and columns to create a specific pattern.
- The outer loop (for ($row=0; $row<7; $row++)) controls the rows of the pattern, iterating from 0 to 6.
- Inside the outer loop, there's another loop (for ($column=0; $column<=7; $column++)) that controls the columns, iterating from 0 to 7.
- Within the inner loop, there's a conditional statement that determines whether to print an asterisk ('*') or a space ( ) based on the position of the current row and column indices.
- The condition checks multiple cases:
- If the row is at index 0 or 6 and the column index is between 0 and 6, inclusive.
- If the sum of the row index and column index equals 6.
- If any of these conditions are met, an asterisk ('*') is echoed. Otherwise, a space ( ) is echoed.
- After printing each row, the code moves to the next line by echoing a newline character ('\n').
- Once all rows and columns are printed according to the pattern, the PHP code ends with a closing PHP tag ?>.
Flowchart :
For more Practice: Solve these Related Problems:
- Write a PHP script to print the letter 'Z' using loops by creating a top and bottom horizontal line with a diagonal line connecting them.
- Write a PHP function to generate a 'Z' pattern with adjustable width and diagonal steps based on input size.
- Write a PHP program to output a 'Z' pattern where the diagonal line is computed via a loop that decrements the starting index on each row.
- Write a PHP script to create a 'Z' pattern using nested loops and manage the spacing so that the shape is proportionate.
Go to:
PREV : Alphabet Pattern 'Y'.
NEXT : PHP Functions Exercises Home.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.