PHP for loop Exercises: Calculate and print the factorial of a number using a for loop
5. Factorial Calculation
Write a program to calculate and print the factorial of a number using a for loop. The factorial of a number is the product of all integers up to and including that number, so the factorial of 4 is 4*3*2*1= 24.
Visual Presentation:
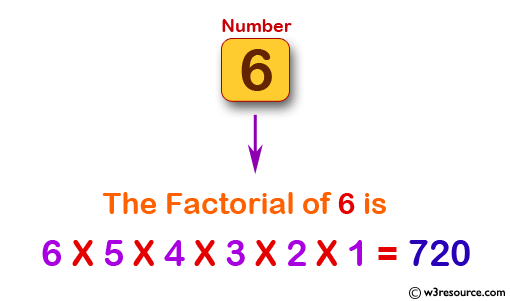
Sample Solution:
PHP Code:
<?php
// Set the value of n to 6
$n = 6;
// Initialize variable x to store the factorial value
$x = 1;
// Loop to calculate the factorial of n
for($i = 1; $i <= $n - 1; $i++)
{
// Calculate factorial iteratively
$x *= ($i + 1);
}
// Print the factorial of n
echo "The factorial of $n = $x"."\n";
?>
Output:
The factorial of 6 = 720
Explanation:
In the exercise above,
- The code begins with a PHP opening tag <?php.
- It sets the variable '$n' to 6, representing the number whose factorial is to be calculated.
- Another variable '$x' is initialized to 1. This variable stores the factorial value.
- A "for" loop calculates the factorial. It iterates from 1 to n-1 ($i=1; $i<=$n-1; $i++), as the factorial of 'n' is calculated by multiplying all positive integers up to n.
- Inside the loop, each iteration multiplies the current value of '$x' by the next integer, starting from 1 up to 'n'.
- After the loop completes, the calculated factorial value is printed using "echo", along with a message indicating the number whose factorial is calculated ("The factorial of $n = $x").
- Finally, PHP code ends with a closing tag ?>.
Flowchart:
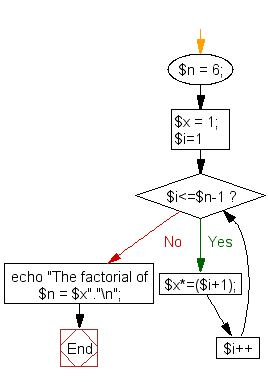
For more Practice: Solve these Related Problems:
- Write a PHP script to calculate the factorial of a given number using a for loop and display intermediate multiplication steps.
- Write a PHP function that computes factorial recursively and compares its performance with a for loop implementation.
- Write a PHP program that calculates and prints the factorial of a number, then outputs the result's number of digits.
- Write a PHP script to compute the factorial of a number and use error handling to manage non-positive inputs.
Go to:
PREV : Construct Symmetric Star Pattern.
NEXT : Two-Digit Decimal Combinations.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.