PHP for loop Exercises: Print all two-digit decimal combination
6. Two-Digit Decimal Combinations
Write a program which will give you all of the potential combinations of a two-digit decimal combination, printed in a comma delimited format.
Sample Solution:
PHP Code:
<?php
// Outer loop for iterating over values of $a from 0 to 9
for($a=0; $a< 10; $a++)
{
// Inner loop for iterating over values of $b from 0 to 9
for($b=0; $b< 10; $b++)
{
// Print the concatenated values of $a and $b followed by a comma and space
echo $a.$b.", ";
}
}
// Move to the next line after printing the entire pattern
printf("\n");
?>
Output:
00, 01, 02, 03, 04, 05, 06, 07, 08, 09, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99,
Explanation:
In the exercise above,
- The code begins with a PHP opening tag <?php.
- It starts with a nested loop structure: an outer loop controls the variable '$a', and an inner loop controls the variable '$b'.
- The outer loop (for($a=0; $a<10; $a++)) iterates over the values of '$a' from 0 to 9.
- Inside the outer loop, there's an inner loop (for($b=0; $b<10; $b++)) that iterates over the values of '$b' from 0 to 9.
- Within the inner loop, the code concatenates the current values of '$a' and '$b' and prints them, followed by a comma and a space.
- This process continues for all combinations of '$a' and '$b', resulting in numbers ranging from 00 to 99 being printed in a pattern separated by commas and spaces.
- After printing the entire pattern, the code moves to the next line using printf("\n");.
- Finally, PHP code ends with a closing tag ?>.
Flowchart :
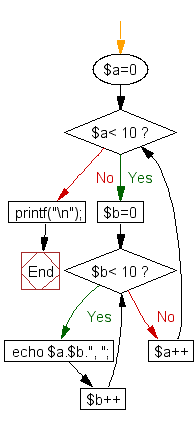
For more Practice: Solve these Related Problems:
- Write a PHP script that generates all two-digit combinations using nested loops and outputs them separated by commas, ensuring a trailing comma is omitted.
- Write a PHP function to build a comma-separated string of two-digit numbers using sprintf() for proper formatting.
- Write a PHP program to loop through numbers 0 to 99, format each as a two-digit string, and join them with a comma, then display the result.
- Write a PHP script to use array_map() on a range from 0 to 99, format each number with leading zeros, and display the output as a comma-delimited list.
Go to:
PREV : Factorial Calculation.
NEXT : Count "r" Characters in a String.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.