PHP for loop Exercises: Count a specified characters in a string
Write a program which will count the "r" characters in the text "w3resource".
Visual Presentation:
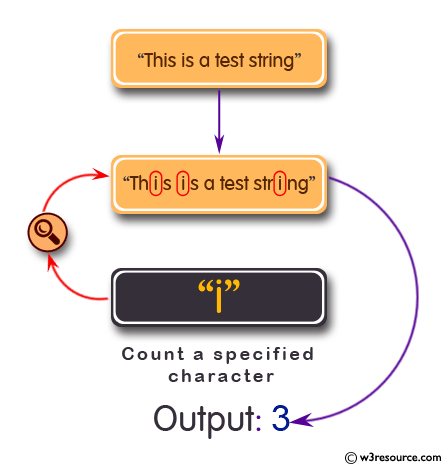
Sample Salution:
PHP Code:
<?php
// Define the text string to search within
$text = "w3resource";
// Define the character to search for
$search_char = "r";
// Initialize a counter variable to count occurrences
$count = "0";
// Loop through each character in the text string
for($x = "0"; $x < strlen($text); $x++)
{
// Check if the current character matches the search character
if(substr($text, $x, 1) == $search_char)
{
// Increment the counter if there's a match
$count = $count + 1;
}
}
// Print the count of occurrences of the search character
echo $count."\n";
?>
Output:
2
Explanation:
In the exercise above,
- The code begins with a PHP opening tag <?php.
- It initializes three variables:
- $text: Contains the text string to search.
- $search_char: Contains the character to count.
- $count: Initializes a counter variable to count the occurrences of the search character.
- The code enters a "for" loop to iterate over each character in the text string. The loop condition is based on the length of the text string (strlen($text)).
- Within the loop, it uses "substr()" function to extract each character from the text string (substr($text, $x, 1)) and compares it with the search character ($search_char).
- If the current character matches the search character, the counter ('$count') is incremented by 1.
- After iterating through all the characters in the text string, the code prints out the count of occurrences of the search character using "echo".
- Finally, PHP code ends with a closing tag ?>.
Flowchart:
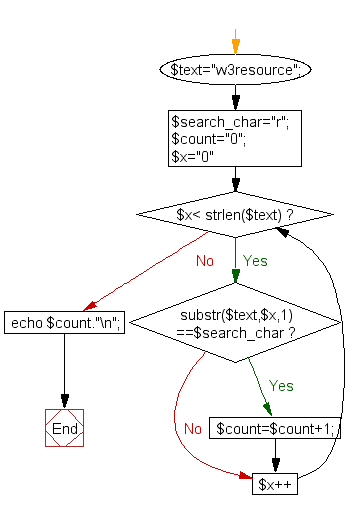
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program which will give you all of the potential combinations of a two-digit decimal combination, printed in a comma delimited format.
Next: Write a PHP script that creates the specific table using for loops. Add cellpadding="3px" and cellspacing="0px" to the table tag.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.