PHP for loop Exercises : Create a table with text in columns using for loops
Write a PHP script that creates the following table using for loops. Add cellpadding="3px" and cellspacing="0px" to the table tag.
Sample Solution:
PHP Code:
<!DOCTYPE html>
<html>
<body>
<table align="left" border="1" cellpadding="3" cellspacing="0">
<?php
// Outer loop for creating rows in the table
for($i=1; $i<=6; $i++)
{
echo "<tr>"; // Start a new table row
// Inner loop for creating cells in each row
for ($j=1; $j<=5; $j++)
{
// Print the multiplication table in each cell
echo "<td>$i * $j = " . $i * $j . "</td>";
}
echo "</tr>"; // End the table row
}
?>
</table>
</body>
</html>
Output:
1 * 1 = 1 | 1 * 2 = 2 | 1 * 3 = 3 | 1 * 4 = 4 | 1 * 5 = 5 |
2 * 1 = 2 | 2 * 2 = 4 | 2 * 3 = 6 | 2 * 4 = 8 | 2 * 5 = 10 |
3 * 1 = 3 | 3 * 2 = 6 | 3 * 3 = 9 | 3 * 4 = 12 | 3 * 5 = 15 |
4 * 1 = 4 | 4 * 2 = 8 | 4 * 3 = 12 | 4 * 4 = 16 | 4 * 5 = 20 |
5 * 1 = 5 | 5 * 2 = 10 | 5 * 3 = 15 | 5 * 4 = 20 | 5 * 5 = 25 |
6 * 1 = 6 | 6 * 2 = 12 | 6 * 3 = 18 | 6 * 4 = 24 | 6 * 5 = 30 |
Explanation:
In the exercise above,
- The code is an HTML document with PHP embedded within it.
- It starts with the declaration of HTML structure, including a <body> tag and a <table> tag to create a table.
- Inside the PHP block, there's an outer "for" loop that runs from 1 to 6 ($i=1; $i<=6; $i++). This loop generates rows (<tr>) in the table.
- Inside the outer loop, there's an inner "for" loop that runs from 1 to 5 ($j=1; $j<=5; $j++). This loop generates cells (<td>) within each row of the table.
- Within the inner loop, it echoes out the multiplication table data using "echo". Each cell contains the result of multiplying the current row number ('$i') with the current column number ('$j'), formatted as $i * $j = result.
- After the inner loop completes for each row, the code echoes out </tr> to close the row.
- After both loops complete, the PHP block ends, and the table HTML tag (</table>) closes.
- Finally, the HTML document is closed with </body> and </html> tags.
View the output in the browser
Flowchart:
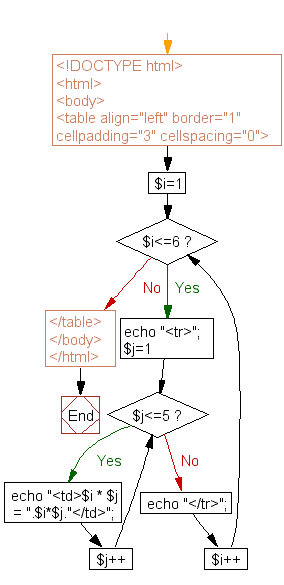
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program which will count the "r" characters in the text "w3resource".
Next: Write a PHP script using nested for loop that creates a chess board as shown below.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.