PHP for loop Exercises : Create a chess board using for loop
PHP for loop: Exercise-9 with Solution
Write a PHP script using nested for loop that creates a chess board as shown below.
Use table width="270px" and take 30px as cell height and width.
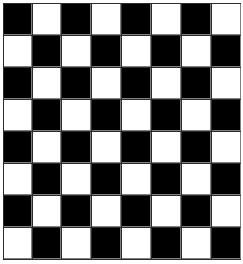
Sample Solution:
PHP Code:
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
</head>
<body>
<h3>Chess Board using Nested For Loop</h3>
<table width="270px" cellspacing="0px" cellpadding="0px" border="1px">
<!-- cell 270px wide (8 columns x 60px) -->
<?php
// Outer loop for rows
for($row=1; $row<=8; $row++)
{
echo "<tr>"; // Start a new table row
// Inner loop for columns
for($col=1; $col<=8; $col++)
{
// Calculate total sum of row and column indices
$total = $row + $col;
// Check if total is even or odd to determine cell color
if($total % 2 == 0)
{
// If total is even, set cell background color to white
echo "<td height=30px width=30px bgcolor=#FFFFFF></td>";
}
else
{
// If total is odd, set cell background color to black
echo "<td height=30px width=30px bgcolor=#000000></td>";
}
}
echo "</tr>"; // End the table row
}
?>
</table>
</body>
</html>
Output:
View the output in the browser
Explanation:
- The code begins with an HTML structure, including a doctype declaration (<!DOCTYPE html>), opening <html>, <head>, and <body> tags.
- Inside the <body> section, there's an <h3> heading stating "Chess Board using Nested For Loop".
- Following the heading, a table is created with a fixed width of 270 pixels (width="270px") and the border attributes set.
- PHP code is embedded within the HTML to generate the chessboard pattern.
- The PHP code starts with an outer "for" loop (for($row=1; $row<=8; $row++)) to iterate through the rows of the chessboard.
- Inside the outer loop, there's an inner for loop (for($col=1; $col<=8; $col++)) to iterate through the columns of each row.
- Within the inner loop, the code calculates the sum of the row and column indices ($total = $row + $col).
- Based on whether the sum is even or odd (if($total % 2 == 0)), the background color of the cell is set to white or black respectively.
- The HTML table cells (<td>) with the appropriate background colors are echoed out within the loop.
- After completing a row, the code echoes out </tr> to close the row.
- After both loops are complete, the PHP code ends, and the HTML structure is closed with closing tags (</table>, </body>, </html>).
Flowchart:
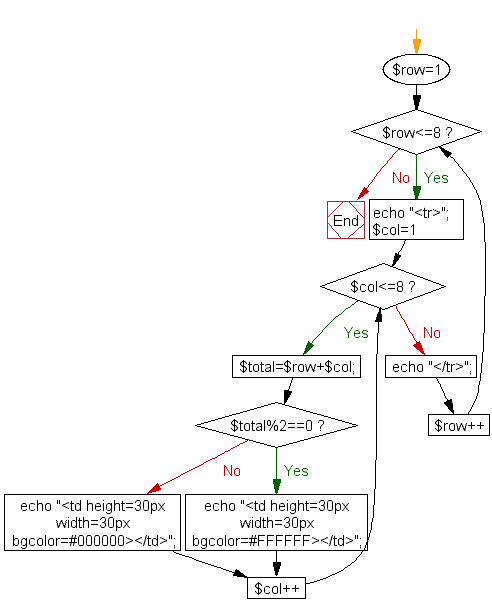
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script that creates the specific table using for loops. Add cellpadding="3px" and cellspacing="0px" to the table tag.
Next: Write a PHP script that creates the specific table (use for loops).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics