PHP function Exercises: Create a function to calculate the factorial of positive a number
1. Factorial Function
Write a PHP function to calculate the factorial of a number (a non-negative integer). The function accepts the number as an argument.
Visual Presentation:
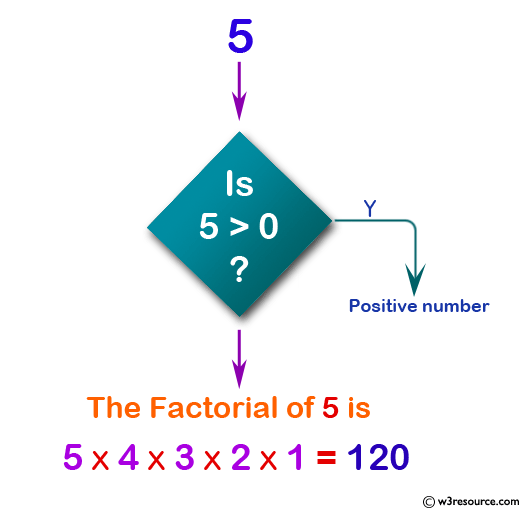
Sample Solution:
PHP Code:
<?php
// Function to calculate the factorial of a number
function factorial_of_a_number($n)
{
// Base case: if n is 0, return 1 (factorial of 0 is 1)
if ($n == 0)
{
return 1;
}
else
{
// Recursive call: calculate factorial by multiplying n with factorial of (n-1)
return $n * factorial_of_a_number($n - 1);
}
}
// Call the function and print the result
print_r(factorial_of_a_number(4) . "\n");
?>
Output:
24
Explanation:
In the exercise above,
- Define a function named "factorial_of_a_number()" which takes an integer parameter '$n'.
- Inside the function, there's a conditional check to handle the base case: if the input number '$n' is 0, the function returns 1, as the factorial of 0 is defined to be 1.
- If the input number is not 0, the function recursively calls itself with the argument '$n - 1' and multiplies the result with '$n'.
- The recursive calls continue until the base case is reached (i.e., '$n' becomes 0), at which point the function returns 1.
- Outside the function definition, the "factorial_of_a_number()" function is called with an argument of 4, which calculates the factorial of 4.
- The result is then printed using "print_r()", followed by a newline character ("\n").
Flowchart :
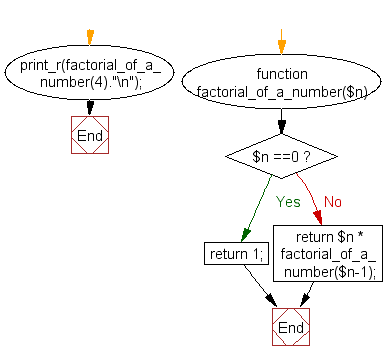
For more Practice: Solve these Related Problems:
- Write a PHP function to compute the factorial of a number using a recursive approach, ensuring proper error handling for negative inputs.
- Write a PHP script to calculate the factorial of a number iteratively and then compare the result with a built-in mathematical formula for large numbers.
- Write a PHP function to compute factorial values for an array of numbers and return the results in an associative array with the original numbers as keys.
- Write a PHP program to compute the factorial of a number using a tail-recursive function and output the recursion depth alongside the result.
Go to:
PREV : PHP Functions Exercises Home.
NEXT : Prime Number Checker.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.