PHP function Exercises: Checks whether a passed string is a palindrome or not
6. Palindrome Checker
Write a PHP function that checks whether a passed string is a palindrome or not?
A palindrome is word, phrase, or sequence that reads the same backward as forward, e.g., madam or nurses run.
Pictorial Presentation:
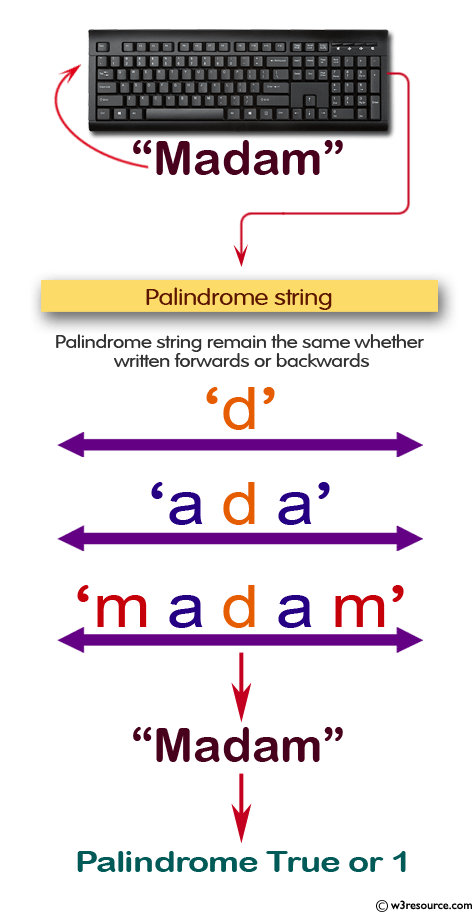
Sample Solution:
PHP Code:
<?php
// Define a function to check if a string is a palindrome
function check_palindrome($string)
{
// Check if the reversed string is equal to the original string
if ($string == strrev($string))
return 1; // Return 1 if it is a palindrome
else
return 0; // Return 0 if it is not a palindrome
}
// Test the function with the string 'madam' and display the result
echo check_palindrome('madam')."\n"; // Output: 1 (true) since 'madam' is a palindrome
?>
Output:
1
Explanation:
In the exercise above,
- The function "check_palindrome()" takes a string '$string' as input.
- Inside the function, it uses the "strrev()" function to reverse the input string.
- It then compares the reversed string with the original string using the equality operator (==).
- If the reversed string is equal to the original string, it returns 1, indicating that the string is a palindrome.
- If the reversed string is not equal to the original string, it returns 0, indicating that the string is not a palindrome.
Flowchart :
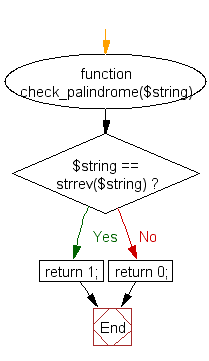
For more Practice: Solve these Related Problems:
- Write a PHP function to determine if a string is a palindrome, ignoring spaces, punctuation, and case differences.
- Write a PHP script to check if a given numeric string is a palindrome and return a boolean result.
- Write a PHP program to check palindromicity recursively, comparing the first and last characters and stripping them away until the string is empty or a single character remains.
- Write a PHP function to test if a phrase is a palindrome by removing non-alphanumeric characters and comparing it to its reverse.
Go to:
PREV : Check All Lowercase String.
NEXT : PHP Regular Expression Exercises Home
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.