PHP Regular Expression Exercises: Checks whether a string contains another string
1. Check if a string contains another string
Write a PHP script that checks whether a string contains another string.
Visual Presentation:
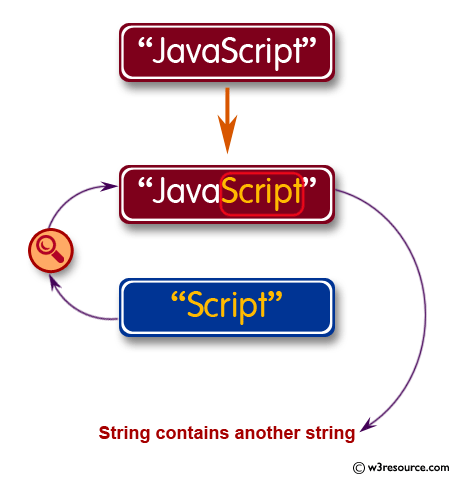
Sample Solution:
PHP Code:
<?php
// Define the regular expression pattern to match 'fox' preceded by a word character and followed by a space
$pattern = '/[^\w]fox\s/';
// Use preg_match function to check if the pattern matches the given string
if (preg_match($pattern, 'The quick brown fox jumps over the lazy dog')) {
// If 'fox' is found in the string, echo that it is present
echo "'fox' is present..."."\n";
} else {
// If 'fox' is not found in the string, echo that it is not present
echo "'fox' is not present..."."\n";
}
?>
Output:
'fox' is present...
Explanation:
In the exercise above,
- A regular expression pattern is defined using the '$pattern' variable. The pattern /[^\w]fox\s/ is used, which matches the word "fox" preceded by a non-word character and followed by a space.
- The "preg_match()" function checks if the pattern matches the given string 'The quick brown fox jumps over the lazy dog'.
- If the pattern matches the string, meaning the word "fox" is found as per the defined pattern, it echoes "'fox' is present...".
- If the pattern doesn't match the string, meaning the word "fox" is not found as per the defined pattern, it echoes "'fox' is not present...".
Flowchart :
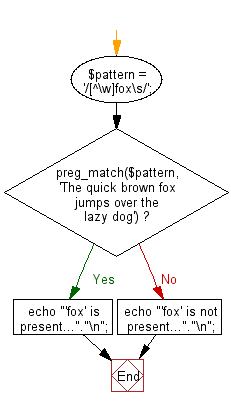
For more Practice: Solve these Related Problems:
- Write a PHP script to find and count the number of occurrences of a substring within a given string, ignoring case sensitivity.
- Write a PHP script to check if any of the strings in an array exist as substrings in a given sentence.
- Write a PHP function to determine whether a given string contains multiple occurrences of a specific word and return their positions.
- Write a PHP script to identify if a keyword appears at the beginning or end of a string, considering both cases and word boundaries.
Go to:
PREV : PHP Regular Expression Exercises Home.
NEXT : Remove the last word from a string.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.