PHP Regular Expression Exercises: Remove the last word from a string
PHP regular expression: Exercise-2 with Solution
Write a PHP script that removes the last word from a string.
Sample string : 'The quick brown fox'
Visual Presentation:
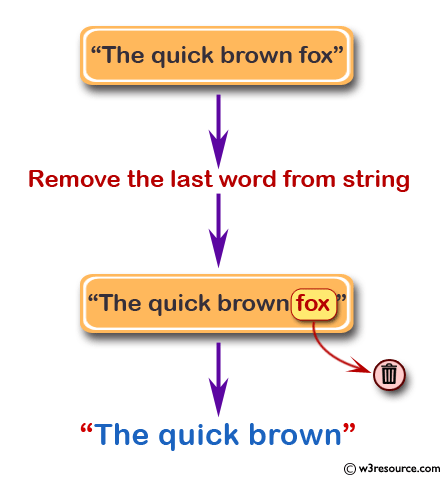
Sample Solution:
PHP Code:
<?php
// Define the input string
$str1 = 'The quick brown fox';
// Use preg_replace function to remove the last word along with its trailing spaces
echo preg_replace('/\W\w+\s*(\W*)$/', '$1', $str1)."\n";
?>
Output:
The quick brown
Explanation:
In the exercise above,
- '$str1' variable stores the input string: 'The quick brown fox'.
- The "preg_replace()" function performs regular expression-based string replacement.
- The regular expression pattern /\W\w+\s*(\W*)$/ is applied to the input string:
- \W: Matches any non-word character (e.g., punctuation).
- \w+: Matches one or more word characters.
- \s*: Matches zero or more whitespace characters.
- (\W*)$: Captures zero or more non-word characters at the end of the string.
- The replacement string '$1' is used to replace the matched pattern with the captured group '$1', which represents the trailing non-word characters.
- The modified string is then echoed.
Flowchart :
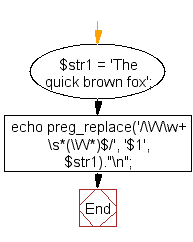
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script that checks if a string contains another string.
Next: Write a PHP script that removes the whitespaces from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics