PHP mail() function
Description
The mail() function is used to send a mail.
Version:
(PHP 4 and above)
Syntax:
mail(to, subject, message, extra headers, additional parameters)
Parameter:
Name | Description | Required / Optional |
Type |
---|---|---|---|
to | Mail address to which you want to send mail | Required | String |
subject | Subject of the mail | Required | String |
message | Message to be sent with the mail. Each line of the message should be separated with a LF (\n). Lines should not be larger than 70 characters. | Required | String |
extra headers | Additional headers like from, CC, BCC. If more than one additional headers are used, they must be separated with CRLF (Carriage return line feed), i.e. new line. | Optional | string |
additional parameters | Additional parameters like - the envelope sender address when using sendmail with the -f sendmail option, can be used using this parameter. | Optional | string |
Return value:
Returns true if the mail is successfully sent, otherwise, it returns false.
Value Type: boolean
Example:
<?php
$to = '[email protected]';
$subject = 'Demo mail ';
$message = 'This is a demo mail. Please reply to make sure the mail communication is okay.';
mail($to, $subject, $message);
?>
Send a simple mail using mail() function
Browse view of the form for sending simple mail in php
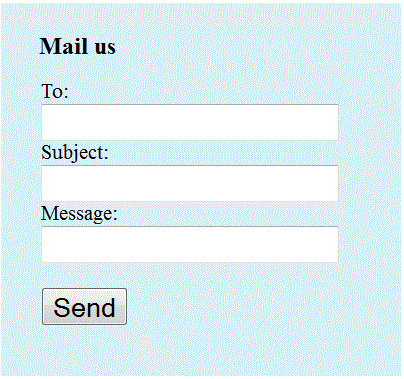
Code:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"><head>
<meta content="text/html; charset=ISO-8859-1" http-equiv="content-type" /><title>send mail in
php</title>
<style type="text/css">
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px solid silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
</style>
</head>
<body>
<div class="mail">
<h2>Mail us</h2>
<ul>
<li><form name="mail" method="POST" action="send.php"></li>
<li>To:</li>
<li><input type="text" name="to" /></li>
<li>Subject:</li>
<li><input type="text" name="subject" /></li>
<li>Message:</li>
<li><input type="text" name="Message" /></li>
<li> </li>
<li><input type="submit" name="submit" value="Send"/></li>
<li> </li>
</form>
</ul>
</div>
</body></html>
Code of the file for handling form (code above) data :
<?php
$to = $_POST['to'] ;
$message = $_POST['Message'] ;
mail( $to, "Mail", $message, );
header( "Location: http://localhost/php/simple-mail.php" );
?>
Send mail with extra headers using mail() function
Browser view of the form for sending mail with extra headers in php
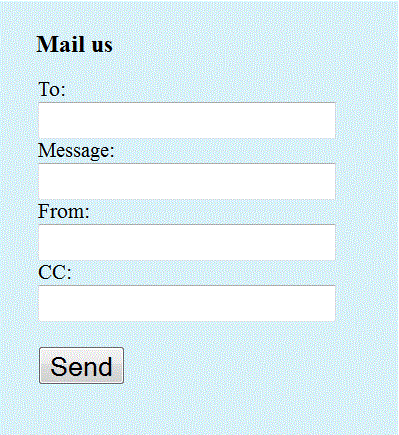
Code:
<!DOCTYPE html PUBLIC
"-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html
xmlns="http://www.w3.org/1999/xhtml"><head>
<meta content="text/html; charset=ISO-8859-1"
http-equiv="content-type" /><title>send mail in
php</title>
<style type="text/css">
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px solid silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;<
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
</style>
</head>
<body>
<div class="mail">
<h2>Mail us</h2>
<ul>
<li><form name="mail" method="POST"
action="send-extra.php"></li>
<li>To:</li>
<li><input type="text" name="to"
/></li>
<li>Message:</li>
<li><input type="text" name="Message"
/></li>
<li>From:</li>
<li><input type="text" name="from"
/></li>
<li>CC:</li>
<li><input type="text" name="cc"
/></li>
<li> </li>
<li><input type="submit" name="submit"
value="Send"/></li>
<li> </li>
</form>
</ul>
</div>
</body></html>
Code of the file for handling form (code above) data:
<?php
$to = $_POST['to'] ;
$message = $_POST['Message'] ;
$from = $_POST['from'];
$cc = $_POST['cc'];
$headers = "From: ".$_POST['from']."\r\n" .
"CC: ".$_POST['cc'];
mail( $to, "Mail", $message, $headers);
header( "Location: http://localhost/php/simple-mail.php" );
?>
We encourage you to replace the value of the parameters used in the above example and test it.
Send mail in PHP with additional parameters
You can pass additional parameters as command line options to the program configured to be used while sending mail. Those said configurations are defined by the sendmail_path configuration. One example of using additional parameter is setting the envelope sender address with -f option.
<?php
mail('[email protected]', 'Demo mail', 'Testing mail communication', null,
'-f [email protected]');
?>
We encourage you to replace the value of the parameters used in the above example and with your's and test it.
Previous: warning_count
Next: PHP secure mail