Python: Get all possible unique subsets from a set of distinct integers
4. Get All Unique Subsets from a Set of Distinct Integers
Write a Python program to get all possible unique subsets from a set of distinct integers.
Sample Solution:
Python Code:
class py_solution:
def sub_sets(self, sset):
return self.subsetsRecur([], sorted(sset))
def subsetsRecur(self, current, sset):
if sset:
return self.subsetsRecur(current, sset[1:]) + self.subsetsRecur(current + [sset[0]], sset[1:])
return [current]
print(py_solution().sub_sets([4,5,6]))
Sample Output:
[[], [6], [5], [5, 6], [4], [4, 6], [4, 5], [4, 5, 6]]
Pictorial Presentation:
Flowchart:
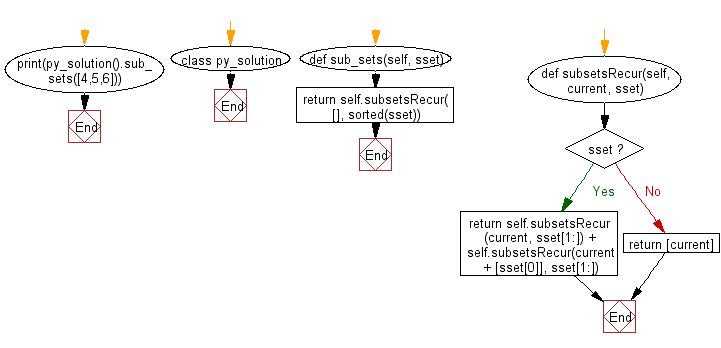
For more Practice: Solve these Related Problems:
- Write a Python class that uses recursion to generate all possible unique subsets of a set of distinct integers.
- Write a Python class that implements an iterative approach to generate the power set of distinct integers and returns it as a list of lists.
- Write a Python class that uses bitwise operations to compute all unique subsets of a given set of integers.
- Write a Python class that sorts and prints all subsets of a set in increasing order of their lengths, using recursion and backtracking.
Go to:
Previous: Write a Python program to find validity of a string of parentheses, '(', ')', '{', '}', '[' and ']. These brackets must be close in the correct order,
for example "()" and "()[]{}" are valid but "[)", "({[)]" and "{{{" are invalid.
Next: Write a Python program to find a pair of elements (indices of the two numbers) from a given array whose sum equals a specific target number.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.